How do I create a News app in React Native?
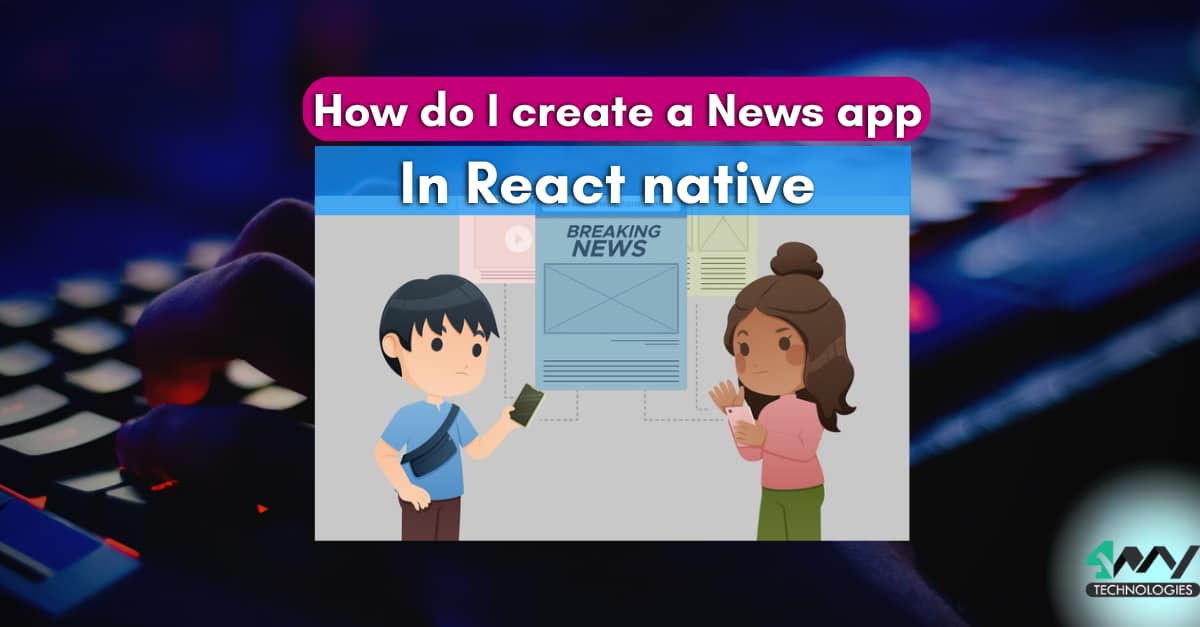
Assuming that you are familiar with the basic concept and utility of the React Native framework, in this tutorial blog, I aim to present step-by-step guidance for creating a News app. Also, apart from building a real-time news app, you will also learn about the use of the API key in getting news from the web server.
So, let's get started.
Note that, you can also get the complete codebase from the linked GitHub repository in case you have already set up the environment, generated the API key and created an RN template, and know how to test the app. However, if you are new in the app development journey and need guidance on each and every step, this blog can be your savior.
Without distracting from the aim of this blog, let's proceed with the first step, i.e. the prerequisites followed by react native developers.
Prerequisites for the News app
The setup procedure
In the first step, you have to install some software and set the Environment. Some of the software are NodeJS, Android Studio, and a code editor for building the code. There are other SDKs that you have to download to build React Native projects. Also, note that we will be using React Native CLI and not Expo CLI. So, get all these software accordingly.
If you are not familiar with the React Native CLI and Expo CLI, then let me tell you that both are the tools used to build React Native apps. The choice of these tools is based on the project requirements.
As I have a reference blog for you to guide you with the steps of the React Native Environment setup, I will not be repeating the same in this blog. Click on the linked article and understand the setup process from the start. Also, you can learn the difference between Expo CLI and React Native CLI from the attached blog.
Create a template in your local drive
Secondly, you need to build a template folder for the current project. So, what you have to simply do is create a folder using a command line. Confused?
Don’t worry. Let me give you the steps.
- Create a folder. Note that it should be in your C drive. Let me name the folder as RNProject.
- Now, go to the RNProject folder and run cmd from this folder.
- Pass the command npx react-native init NewsApp --version 0.68.1 in the cmd that you opened from the RNProject folder. Click on the enter key.
- Your template with the name NewsApp has been created in the RNProject folder. You will notice that all the basic files required for a React Native project are embedded inside the template.
Project setup configuration: Axios installation
Thirdly, you have to install the axios library and link it to your project. If you are not aware of the usage of this library then axios is a JS-based library. You can use it in your project to execute either HTTP requests or XMLHttp requests where the former request is from node.js and the latter request is from the browser. It provides support to client sider servers protecting user data against Cross-site Request Forgery.
Here, in this project, axios library is used to perform the GET request to a web server.
For its installation, you have to run the command npm i axios on the project terminal.
Generate the API key for the News App
Lastly, you have to get the API key. For this step, you have to visit the linked site and register for the API key. After completing the registration, your API key will be created. This API key will allow the program to get the current news from the server through request authentication.
You have to use this API key in your codebase. We will discuss this in the following codebase segment.
Creating files and embedding codebase
For this project, you need to create two files in .js format. These are as follows.
- NewsRender.js
- App.js
Let's start coding.
NewsRender.js
Open the template folder news from the VS code editor. Create a ‘components’ folder in it. Now create a file named ‘NewsRender.js’ in the ‘components’ folder.
Let's see what codebase we need to embed in the NewsRender.js file.
1
2
3
import { StyleSheet, Text, View ,Dimensions, ScrollView, Image } from 'react-native'
import React,{useEffect,useState} from 'react'
import axios from 'axios'
The code imports components from the react-native, react,
and axios
.
The StyleSheet, Text, View, Dimensions, ScrollView
, and Image
components are imported to frame the user interface.
The axios and React libraries are also imported. The useEffect and useState are the React Native hooks that will be used to manage the state of the application.
The useEffect hook is used to perform operations like making an API call whereas the useState hook is used to store data in the app’s state.
The library axios is imported to make HTTP requests to a server.
1
2
3
4
const NewsRender = () => {
const Width=Dimensions.get('window').width
const [dataWidth, setDataWidth] = useState()
const [getData,setGetData]=useState(null)
This code uses React Hooks to render news from an API call.
This code uses Dimensions.get()
method to get the width of the window and store it in the Width variable.
Later, it creates two state variables, dataWidth, and getData.
The initial value of the dataWidth variable is set to empty and the getData variable is initialised to null.
The useState()
hook allows the dataWidth
and getData
variables to be updated and accessed throughout the component.
1
2
3
4
5
6
7
8
9
10
const ApiKey="***********253659dfc6"
useEffect(() => {
if (Width < 500) {
setDataWidth(Width/1.1)
}
else if(Width>500 && Width<800){
setDataWidth(Width/3-20)
}
}, [Width])
You can notice that I have hidden some parts of the APIKey with *s. It is because hackers can use the API key to get important credentials that may result in data abuse. You can create your own API key from the site mentioned in the last segment of the prerequisite section.
In this code, the useEffect hook is used to check the value of the variable Width. If the value of Width is less than 500, it will set the value of the variable DataWidth to be equal to Width divided by 1.1. If the value of Width is greater than 500 and less than 800, it will set the value of DataWidth to be equal to Width divided by 3 minus 20.
The last line of this code syntax uses the JavaScript spread operator. It is used to expand an array into its individual elements. In this instance, the array [Width] is spread into the object that follows it.
1
2
3
4
5
6
7
8
9
10
11
const NewsAPI=()=>{
axios({method:'get',
url:`https://newsapi.org/v2/top-headlines?category=technology&language=en&apiKey=${ApiKey}`,
headers:{}
}).then(response=>{
console.log(response.data)
setGetData(response.data.articles)
}).catch(error=>{
console.log(error)
})
}
The code syntax uses the NewsAPI to make an API call and get the top headlines in the technology category. It also uses the Axios library to make the API call. As you can notice that the API key is stored in the placeholder ${ApiKey}.
The headers object is empty as no headers are needed for this API call.
The .then() method is used to handle the response from the API call. The response will be passed as an argument to the callback function inside the .then() method.
The response from the API call is logged to the console and then stored in the setGetData variable.
However, if there is an error, the error will be logged into the console.
1
2
3
useEffect(()=>{
NewsAPI()
},[])
In this code, the useEffect hook is used to call the NewsAPI function when the component is rendered. The empty array is used as the second argument to the useEffect hook. It ensures that the NewsAPI function is only called once when the component is rendered.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
return (
<ScrollView>
<Text style={styles.Text_News}>News</Text>
{getData && getData.map((val,index)=>
<View style={{width:dataWidth,height:280,alignSelf:'center',
marginTop:10,elevation:5,borderWidth:1}} key={index} >
<Image source={{uri:val.urlToImage}} style={{width:dataWidth,height:150,marginTop:10,resizeMode:'contain'}}/>
<Text style={styles.text_name}>{val.author}</Text>
<Text style={styles.title_name}>Title:- {val.title}</Text>
<Text style={styles.data_type}>Date:- {val.publishedAt}</Text>
</View>
)}
</ScrollView>
)
}
This code renders a ScrollView
with a Text element ‘News’ and a View element. Here, the text element applies a style using the style object Text_News. You can find the styling applied to it later in the styling part.
The map()
method used in the syntax is used to loop through an array called getData and also render a div for each item in the array. The map() method takes two arguments, val and index, which represent the value and index of the current item in the array.
The View element further contains an Image and three text elements. The View element also has a specific value to its width, height, alignSelf, marginTop,
elevation, and borderWidth properties.
The key property is set to the value index. key={index} sets a key-value pair in an object. The key is "index" and the value is whatever is assigned to the variable "index". This is a way to store data in an object.
The image source has the value of the urlToImage property of the val object. The style of the image has a width of dataWidth, a height of 150, a margin of 10 at the top, and a resize mode of ‘contain’.
The last two text elements display ‘Title:-’ and ‘Date:-’ on the screen.
styles.text_name, styles.title_name and styles.data_type are the styling applied to the object text_name, title_name and data_type respectively.
val.publishedAt
refers to a value (stored in a variable named val). It has a property named publishedAt
. This value can be an object, such as a JSON object returned from an API. It contains information about the content (here, news article) and its publication date.
Similarly, val.title
and val.author
have a property title and it has the information about the title and author respectively of the content.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
export default NewsRender
It exports NewsRender as a component so that it can be used in other files.
const styles = StyleSheet.create({
Text_News:{
color:'#000000',
alignSelf:'center',
fontSize:20
},
text_name:{
fontSize:16,
marginHorizontal:20,marginTop:5,
color:'#000000'
},dec_Text:{
height:38,width:320,
alignSelf:'center'
},title_name:{
fontSize:14,
marginHorizontal:20
},data_type:{
marginTop:3,
marginHorizontal:20
}
})
This code frames StyleSheet
object styles
. It contains styling properties.
The Text_News property sets the color to black, aligns the text to the center, and sets the font size to 20.
The text_name property sets the font size to 16, adds a margin of 20 horizontally and 5 vertically, and sets the color to black.
The dec_Text property sets the height to 38 and width to 320 and aligns the text to the center.
The title_name
property sets the font size to 14 and adds a margin of 20 horizontally.
The data_type
property adds a margin of 3 vertically and 20 horizontally.
For the App.js file, we will consider the following codebase.
App.js file
You don't have to create this file as you have done for the NewsRender.js file. You can find this App.js file already stored in your template. What you need to do is create the codebase for the file and call the NewsRender component.
So, let's start.
1
2
3
import { StyleSheet, Text, View } from 'react-native'
import React from 'react'
import NewsRender from './components/NewsRender'
You will already find that the codebase in your App.js file has imported the StyleSheet, Text, View, and React from the relevant libraries.
You only need to use the import statement to import NewsRender
from ./components/NewsRender
. It is the path in which you have stored your NewsRender.js
file.
1
2
3
4
5
6
7
const App = () => {
return (
<View>
<NewsRender/>
</View>
)
}
Now you have to render the View component and wrap the <NewsRender/> in it.
The NewsRender component is a custom component that will display news content.
export default App
const styles = StyleSheet.create({})
This codeline is already stored. The first line exports the App component and the second line is a way to use the StyleSheet component.
We are done with creating the codebase.
To run the program on a device
Here, the device that we will consider is the emulator.
Follow the steps mentioned below.
- Go to the Command prompt.
- Type cd news, which will take you to the template folder.
- Run
npm install
and thennpx react-native run-android
. It will start the emulator on your system. - Wait until the bundling process is done.Consider image 1 as the output for the news app on the emulator.
Consider image 1 as the output for the news app on the emulator.
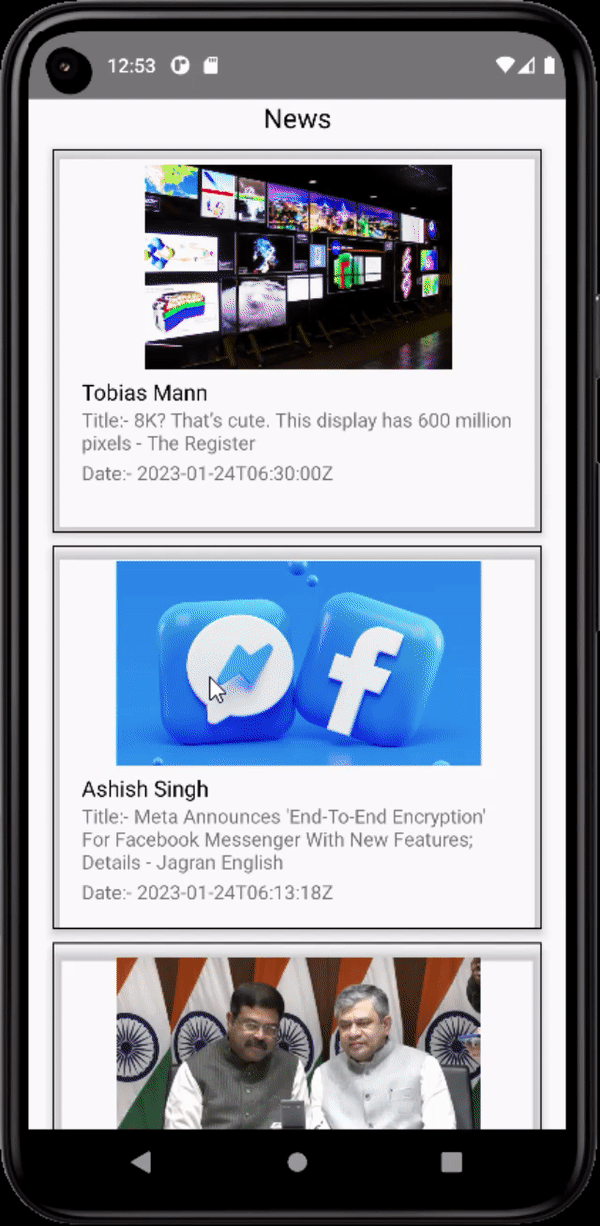
Image 1
Final Notes
Creating an app is not too difficult if you have a clear concept of the fundamentals of the chosen app development framework. Here, it is React Native. So, I would suggest you set up the environment, make the template and install relevant libraries. Creating a codebase becomes much easier when you are familiar with its algorithms. So, start practicing.

A science graduate who has a keen interest to lean about new technologies and research area. With an experience in the field of data analytics and content writing, she aims to share her knowledge among passionate tech readers.