React Native SectionList Explained- A Tutorial with Examples
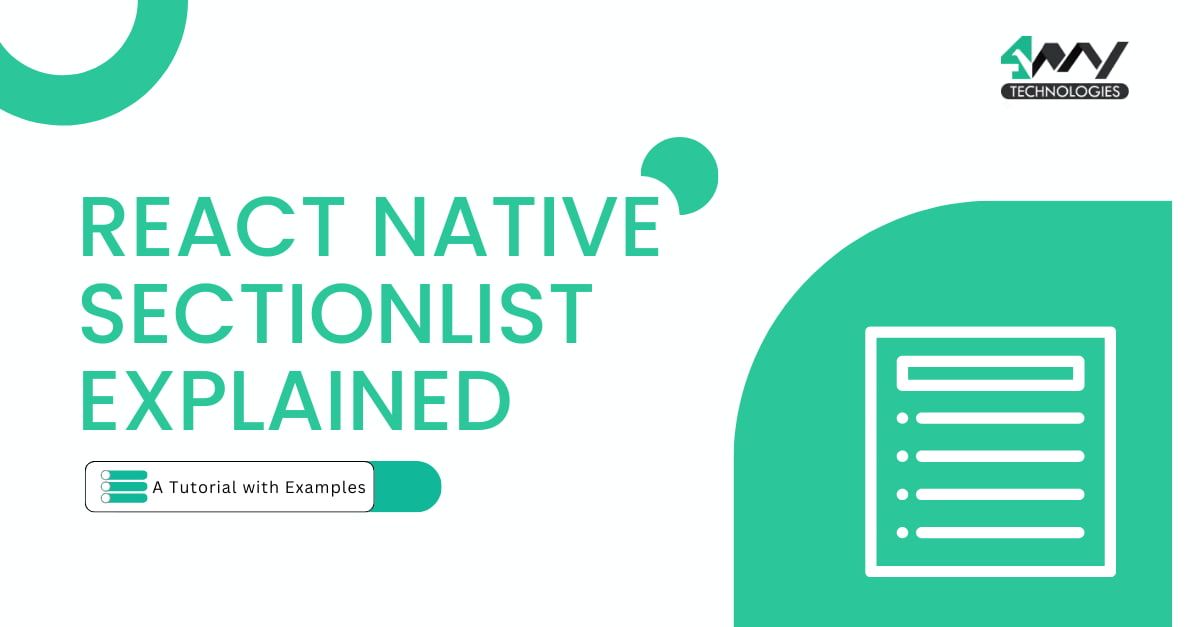
Managing lists might seem ordinary, but what if there was a dynamic way to transform this everyday function into an engaging experience? React Native SectionList does that. It is because of the broad features available to the framework that experts of a React Native app development company prefer it. With real examples, this article is curated to empower you to create a visually engaging list of views. Getting insights from this tutorial, you can further customize the feature.
So, let’s check all the steps and the coding part.
Pre-requisite Consideration
Why this section? This is required for every project that uses React Native. First, you have to set up the environment, and the next thing that you have to do is create a folder. So, here is the step-by-step guide.
The Environment Setup
When you consider React Native, there are two tools that you can choose from. Either React Native CLI or Expo CLI. For this project, we have used the React Native CLI. Apart from this CLI, you need to install some other software. They are Android Studio and Visual Studio Code Editor. Also, install npm globally into your system.
Don’t forget to get the Node.js installation. Node.js is used to manage the installed packages efficiently through npm.
Make sure to follow the detailed guidelines provided by React Native for the specific version you are working with. For further assistance, you can check the blog ‘How to set up the React Native Development Environment’.
A Separate Folder for the Project
Where to store the project asset? You need a definite folder for this. Isolate the project in its folder. Hence, you can ensure a clean workspace without interfering with other system files. It allows for more effortless navigation, better version control, and streamlined collaboration.
How can you create a folder? It’s different from the basic process. All you need to do is create a folder the way you usually do. Open the terminal from the folder and pass a command: npx react-native init SectionList --version 0.72.3.
This will build all the basic files needed for a React Native project.
Note: SectionList
is the project’s name, and 0.72.3 is the React Native version we have used.
Let’s Start Coding for the Project
Here, we will work on the App.js file. Check the complete source code in the GitHub repository.
Importing the Required Components
import React from 'react';
import { SectionList, StyleSheet, Text, View } from 'react-native';
The code snippet is the initial point of a React Native project. It uses the 'SectionList' component.
React is imported to use the entire React library. SectionList is taken to build a list view as sections. It is necessary to present data in a categorized way. 'StyleSheet', 'Text', and 'View' are additional components used for overall layout and styling.
A Task List Structure
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
export default function App() {
const newTaskData = [{
title: "New Tasks",
data: [
{
id: "1",
task: "Buy groceries"
},
{
id: "2",
task: "Feed Cat"
},
{
id: "3",
task: "Sleep for 3 hours"
},
{
id: "4",
task: "Water Plants"
},
{
id: "5",
task: "Drink Water"
},
]
}];
A function named 'App' is specified in the code. There is a variable 'newTaskData' with task list.
'newTaskData' is an array with one object in it. The title "New Tasks" is defined in the object. A data key is defined as an array. It holds a list of tasks. Each task has an id and a description like "Buy groceries" or "Feed Cat." Five tasks are defined in total.
Organizing Completed Tasks
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
const completedTaskData = [{
title: "Completed Tasks",
data: [
{
id: "6",
task: "Make a section list tutorial"
},
{
id: "7",
task: "Share this tutorial"
},
{
id: "8",
task: "Ask doubt in the Comments"
},
{
id: "9",
task: "Subscribe to logrocket"
},
{
id: "10",
task: "Read next Article"
},
{
id: "11",
task: "Read next Article 2"
},
{
id: "12",
task: "Read next Article 3"
},
{
id: "13",
task: "Read next Article 4"
},
{
id: "14",
task: "Read next Article 5"
},
{
id: "15",
task: "Read next Article 6"
},
{
id: "16",
task: "Read next Article 7"
},
{
id: "17",
task: "Read next Article 8"
},
{
id: "18",
task: "Read next Article 9"
},
{
id: "19",
task: "Read next Article 10"
},
]
}];
This code structures the completed tasks. It has an array called 'completedTaskData'. Further, it defines the title 'Completed Tasks' and a list of individual tasks.
Overall, it creates a list of finalized tasks. It organizes tasks like making a tutorial, sharing it, and reading articles into a category called "Completed Tasks." It is a clean way to track the tasks achieved.
Organizing New and Completed Items with SectionList
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
return (
<View style={styles.container}>
<SectionList
sections={[...newTaskData, ...completedTaskData]}
renderItem={({item})=>(
<Text style={styles.taskItem}>{item.task}</Text>
)}
renderSectionHeader={({section})=>(
<Text style={styles.taskTitle}>{section.title}</Text>
)}
keyExtractor={item=>item.id}
stickySectionHeadersEnabled
/>
</View>
);
This syntax is meant to display assigned tasks on the screen. It is presented in the form of sections. There are two sections: "New Tasks" and "Completed Tasks."
The outer <View> acts like a container that wraps everything inside. It has a style that helps in styling what's inside.
<SectionList> is a unique component that displays items listed in different sections. In the present case, we have two sections: new and completed tasks. The 'renderItem' takes each task (like "Buy groceries") and specifies how to show it on the screen. 'renderSectionHeader' is used to display section headers.
The 'keyExtractor' helps track each task in the list using the task's unique ID. It is like giving a name tag to each task. The 'stickySectionHeadersEnabled' is a feature applied to keep the section headers visible at the top of the screen as users scroll down. It's like a sticky note.
Styling the Section List
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#eafffe'
},
taskItem:{
padding: 10,
marginVertical: 15,
fontSize: 16
},
taskTitle:{
backgroundColor: "#ffffff",
fontSize: 20,
fontWeight: "bold",
padding: 10,
elevation: 4,
margin: 10,
marginBottom: 0,
borderRadius: 10
}
});
This section defines styles for different elements within the project. The 'container' sets the background color. The 'taskItem' styles individual tasks with padding and font size, and the 'taskTitle' formats the titles with specific background color, font size, weight, and padding.
Let’s Run the Program
As we have completed the coding part, you have to check if it is running properly. For this, follow the simple steps.
- Go to your project folder and run the terminal.
- Run two commands: npm
install
and thennpx react-native run-android
.
Wait for some minutes until the code successfully runs on your emulator.
Here is the gif of the build shown below.
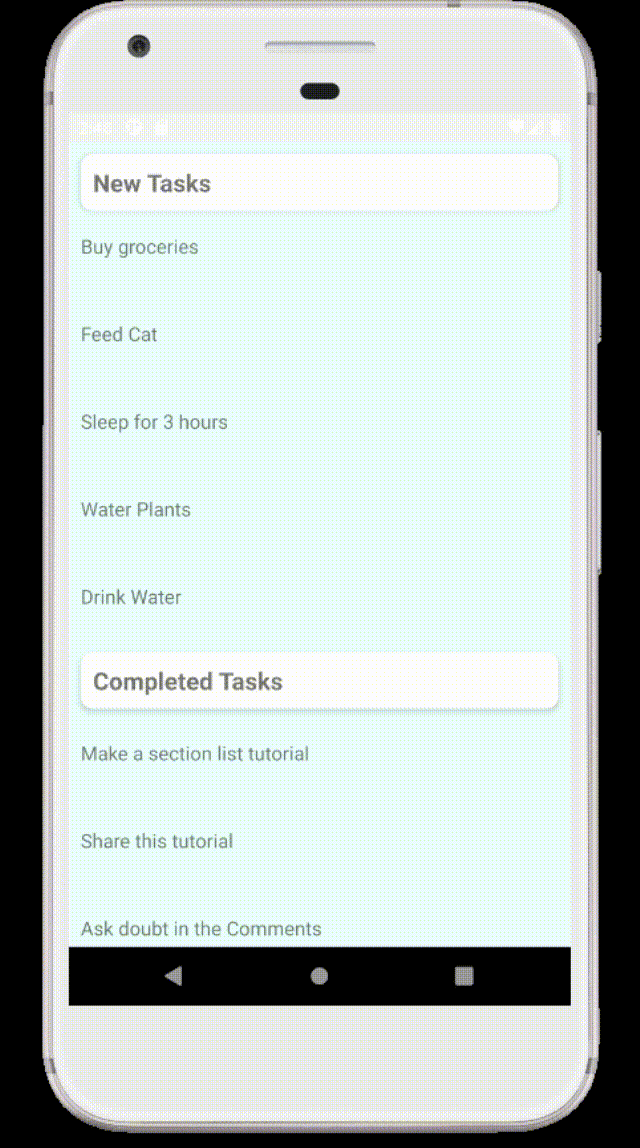
As you can notice, there are two sections created. The New Tasks and The Completed Tasks.
Final Thoughts
That's a wrap on the React Native SectionList project! Now, with this feature added to your app, you can organize tasks like a pro and make your app look more functional. Thus, this tutorial offers an accessible way to start with React Native's SectionList. Happy coding.

A science graduate who has a keen interest to lean about new technologies and research area. With an experience in the field of data analytics and content writing, she aims to share her knowledge among passionate tech readers.