How to make a GET request to an API from a React Native app
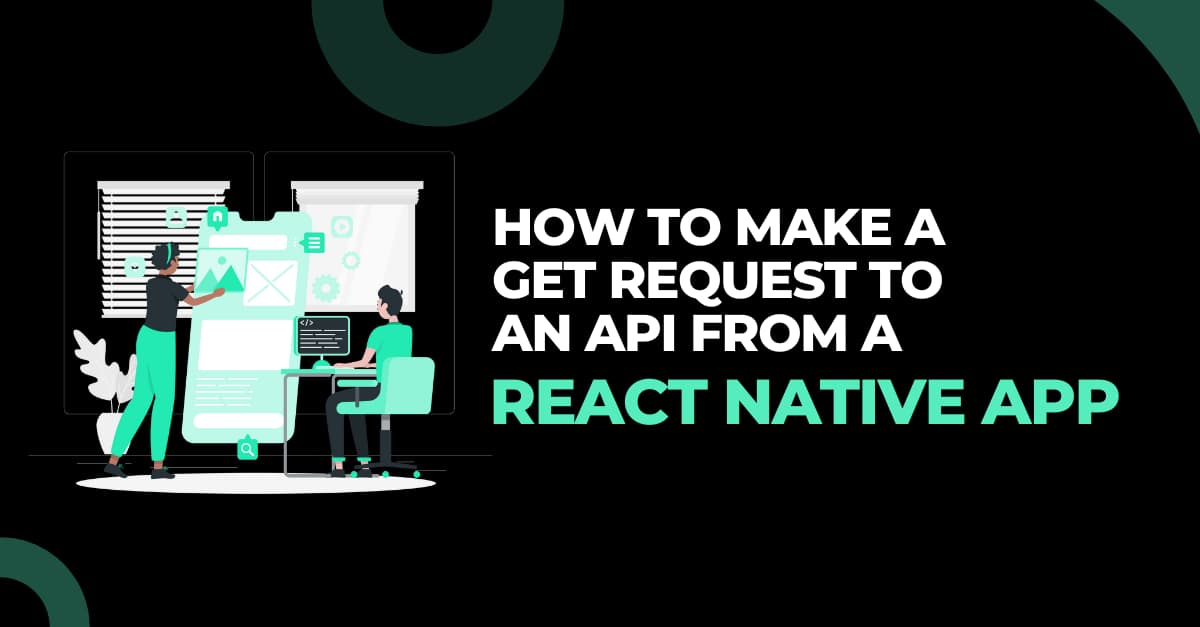
Making requests to an API is a quite useful process to fetch services or data from other applications. With React Native app, you can easily make a GET request to an API. This article will make you familiar with all the terminology along with step-by-step tutorials to making a GET request to an API from a React Native app.
GET Method
Before getting into the concept of the GET method, you should become familiar with the ‘GET Request’.
What is a GET request?
GET request is the method of retrieving information or data from a server with a specific URL. Such GET requests do not have any impact on the collected data. As far as GET is concerned, it is the most common technique for making HTTP requests.
To make the concept simpler, think it like, you undertake the GET method to obtain data from a particular server in an organized manner. In a daily example, you can think of GET requests as the order that you place for getting something like apparel, food, or any other stuff. In this case, you are the application that is making the GET request to fetch the order from a specific server such as the food or apparel providers. However, you cannot make a direct request. Here, starts the role of the middleman, that is the API or Application Programming Interface. In this general example, the online e-Commerce platform is the API.
API calls
You won’t get the idea of API calls if you are unaware of the concept of an Application Programming interface.
What is An API?
API is defined as the procedures, tools, and protocols that support interaction or connection between two applications. API is one of the crucial parts required in program creation and also it speeds up the process. You can consider API as the software through which you make a certain request to some web servers.
API has made the work process of a developer much easier. With API, they don’t have to indulge in repeated and complex coding thereby saving a lot of time and focusing more on the core tasks.
API calls are the orders or requests. If you, as a client, ask for some information from the server, you have to make an API call.
Now let’s come to the tutorial steps of how you can make GET requests to an API from a React Native application. You can check the entire code used in theGithub repository.
Key terms used and their usage
1. ActivityIndicator-ActivityIndicator
is a component in React Native which is imported into a codebase to show a loading indicator on the screen. Refer to the circular loading display in image 1.
2. useEffect- useEffect
is a hook using which you ask React that the component in which this hook has been used needs to perform a task after the render. It helps to increase the responsiveness of the app.
3. useState-
It is a kind of Hook with which you connect React state to different functional components. In a project, you have to import useState from ‘react’.
4. Console.log-
It is a JavaScript function used for the purpose of debugging. It also allows you to display defined variables on the directed screen.
5. ScrollView- ScrollView
is a scrolling container that you can import from react native. It contains different views and components. The scrollable items are heterogeneous, which implies that users can scroll both horizontally and vertically.
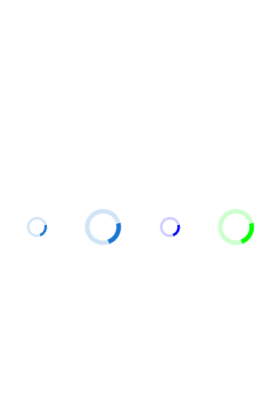
Before starting with the steps of making a GET request, you have to develop an app with React Native development company. If you are struggling with the process, check the article to get detailed step-by-step guidance. You can choose either of the tools React Native CLI or Expo CLI. Since we have used a demo expo app, start with the same.
Codelines and a detailed interpretation
You need to start making GET requests with the following codeines. Although you can get the entire code in the GitHub repository, I will explain every segment of the code line for better understanding.
1
2
3
import React, {useEffect, useState} from 'react';
import {ActivityIndicator, ScrollView, Text, View} from
'react-native';
- I have imported
ScrollView, Text, View
, andActivityIndicator
Components from React Native. - I have also imported
useState
anduseEffect
from React.
1
2
3
4
const App = () => {
const [isLoading, setIsLoading] = useState(true);
const [response, setResponse] = useState(null);
const [error, setError] = useState(false);
- The above code line is a function that contains objects of different properties
response, error,
andisLoading.
- With the useState function, you can set a state variable for the individual property. It even returns functions that update these variables on calling.
- The variable
isLoading
will be true when the page starts loading and will turn to false when it is done loading. - In the initial phase, the variable response is set as null. When the user submits an API URL with the codebase, the state variable of
response
changes. - The variable
error
will be false until an error occurs on submitting data.
1
2
3
4
5
6
7
8
9
useEffect(() => {
fetch('https://covid-19-statistics.p.rapidapi.com/regions', {
method: 'GET',
url: 'https://covid-19-statistics.p.rapidapi.com/regions',
headers: {
'X-RapidAPI-Key': '5ad52ae891msha57664fb78d9e38p1be7edjsn79ae240f9e3a',
'X-RapidAPI-Host': 'covid-19-statistics.p.rapidapi.com',
},
})
- Using the ‘GET’ method, the code line starts with fetching data from the URL https://covid-19-statistics.p.rapidapi.com/regions.
- The code sends requests to the URL with specific headers, including definite RapidAPI-Key and RapidAPI-Host. Since creating an API is a backend task, I have taken a sample API URL from the site Rapid API. While taking any API URL from this site, you also have to take the API key and the API host.
- Here, we have used fetch() to make GET requests to the considered RapidAPI services.
- Since we are concerned about making GET requests to the API, this codebase will display JSON responses on the app screen. It will show covid related data on specific regions.
1
2
3
4
5
6
7
8
9
10
11
12
.then(response => response.json())
.then(data => {
console.log('data', data);
setIsLoading(false);
setResponse(data);
})
.catch(error => {
console.log('something failed miserably');
setIsLoading(false);
setError(true);
});
}, []);
- With the
.then(response => response.json())
, the code calls the data in json response. - The variable
setIsLoading
determines if the chosen URL is loading or not. The other variablesetResponse
is created to hold the data from the made GET request. - We have considered other variables
setEerror
as true andsetIsLoading
as false if something error occurs in calling the API. In this state, the code will log the text ‘something failed miserably’ in the Metro bundler. - I have framed the codebase in a way that it will check the status of the server call and then will show
setResponse
with the data andsetIsLoading
as false.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
return (
<View>
{isLoading ? (
<ActivityIndicator size={30} />
) : error ? (
<Text>ERROR OCCURED</Text>
) : (
<ScrollView>
<Text>{JSON.stringify(response)}</Text>
</ScrollView>
)}
</View>
);
};
export default App;
- I have used
<View></View>
to design the server response and design the operation of theActivityIndicator.
- The
<View></View>
components used in the code linescontain three variables ofisLoading, error,
and displayingJSON
data. - I have set the size of the ActivityIndicator as 30 pixels.
- The code line states that if the data from the server is loading, it will show the circular loading motion, or else it will display the text ‘ERROR OCCURRED’ on the screen.
- The component <ScrollView> is used to display JSON data in string form. With this component, you can scroll down to all the data contained in the chosen server.
Final thoughts
Calling API or making GET requests from a server makes the data collection process much easier. Although the process seems to be somewhat tricky, I have simplified each code line with its easy interpretation. This will not only help you to acquire knowledge on the concept of Making GET requests from React Native app but also you can create your own customized API call from the URL you would prefer.

A science graduate who has a keen interest to lean about new technologies and research area. With an experience in the field of data analytics and content writing, she aims to share her knowledge among passionate tech readers.