How to do hashing in React Native?
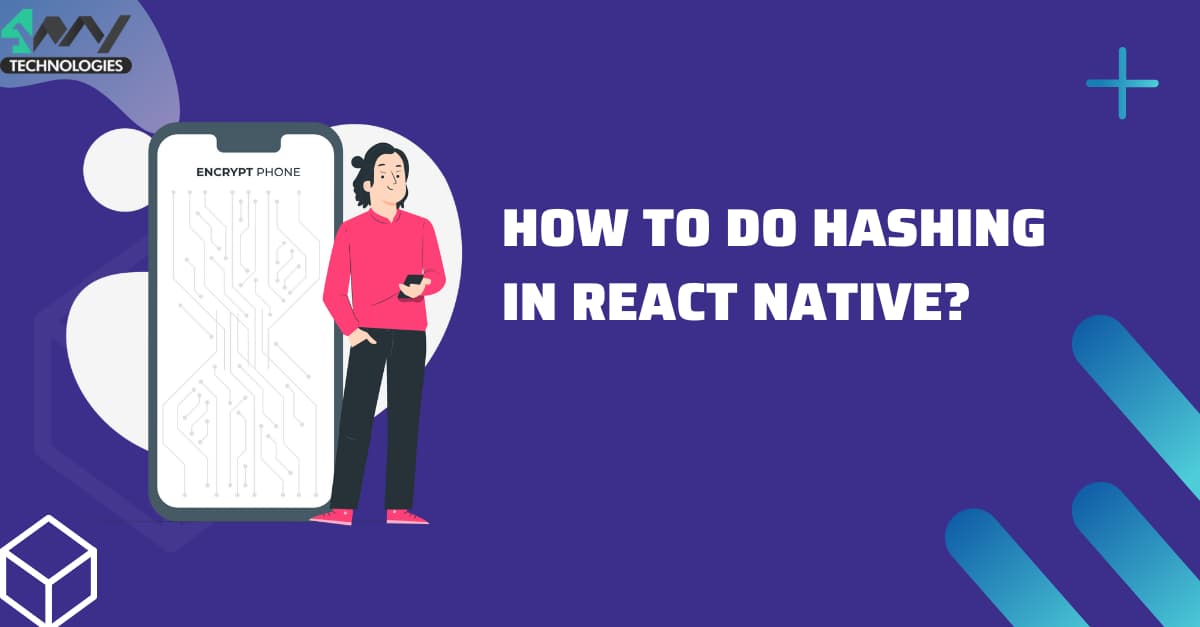
Hashing is a well-known technique used to secure the storage and transmission of data required in software development. In React Native, hashing can be used to store passwords, authenticate users, or validate data integrity. To use hashing in React Native, you need to use third-party libraries that will help you create the hashes. Still, trying to figure out where to start? Don’t worry. This blog, brainstormed by the editors of React Native app development company will give you a detailed insight into how to use these libraries and learn the functionality of hashing in React Native.
Before going further, let’s discuss its use case in detail.
What is Hashing and What are its use cases?
Hashing is the process of transforming data of any size or type into a fixed-size output, called a hash. The hash represents the original data in a unique way that cannot be reversed or easily duplicated. It is a one-way process, meaning it is nearly impossible to recreate the original data from the hash.
The use cases for hashing are given as follows.
- Hashing is generally used to store passwords securely.
- It can be used to validate that data has not been tampered or altered with.
- It is used to create digital signatures, which are further considered to detect the authenticity of a message or document.
- It is used to index data for faster search. With hashtables, you can store data; allowing for quick retrieval using the unique hash key.
Let’s find out if we have to perform any prerequisites before actually coding.
Yes, you have to undertake the following mentioned tasks.
Environment setup
If you have not installed the relevant software required for React Native projects, Environment setup is an important task that you need to perform. Based on the OS, we will use for the project, we need Android Studio, Node, JDK, VS code editor, and React Native CLI. You can check the linked article for further details.
Note, that this is a one-time process. If you have already set up the environment, you don’t have to repeat this step. Also, if you want to go with the other OS, then the setup process will differ.
A template folder for the project
For this part, you have to create a container-like element where you can store your codebase. Follow the below-mentioned steps to build this template folder.
- Create a new folder in your dev system.
- Run cmd from this folder or open a terminal from this created folder.
- Type npx react-native init Hashing --version 0.71.3.
- Click on the Enter key and your template will be created.
Now, you can get the third-party plugin and create the codebase.
Installing react-native-hash
React Native supports several built-in components; however, you cannot find any hashing library. So, in this case, you have to use a third-party library like react-native-hash. To use this library, you need to install it using the npm command.
Run npm install react-native-hash --save on your project terminal. Here, it is ‘Hashing’. As you have installed the library, you can import components such as JSHash, JSHmac, and CONSTANTS
.
Understanding the Project Codebase: Guidelines for code consistency
I understand if you are only looking for the code source. Here, it is. For other readers, who want to decode the basis of the project, stay with us till the end of the project.
Creating the HashToken.js folder
For this older, you may create a ‘Component’ folder under which add this .js folder. Let’s find out the codebase for this project.
1
2
3
import { StyleSheet, Text, TextInput, TouchableOpacity, View,} from 'react-native'
import React, {useState} from 'react'
import { JSHash, JSHmac, CONSTANTS } from "react-native-hash";
These are the components that the code imports. React and useState are taken from the ‘react’ library. StyleSheet, Text, TextInput, TouchableOpacity, and View components are imported from the ‘react-native’ library.
Lastly, it imports JSHash, JSHmac, and CONSTANTS from the third-party plugin namely react-native-hash.
JSHash is used to compute hashes with hash algorithms like SHA-256, SHA-384, and SHA-512. JSHmac is a function that uses Hash-based message authentication codes to compute hashes and CONSTANTS are used by the hashing functions like supported hash algorithms.
1
2
3
4
5
const HashToken = () => {
const [text,setText]=useState()
const [stringText,setString]=useState()
const [secretKey,setSecretKey]=useState()
const [hmac256,setHmac256]=useState(false)
This snippet defines a functional component HashToken. It uses the const keyword and an arrow function syntax.
The component uses the useState hook to define four state variables: text, stringText, secretKey, and hmac256.
Here, the text is used to make space for the input text that will be hashed. The stringText is for the input text that will be used as a string for JSHmac function.
secretKey holds the secret key, which is used to generate the Hash-based message authentication codes (HMAC). Lastly, hmac256 comprises a boolean value, which indicates whether the algorithm associated with HMAC is used for generating the hash is HMAC-SHA256.
The initial values of all these state variables are set as undefined, except for hmac256, which is false.
The purpose of hmac256 is to allow the user to select the HMAC algorithm they prefer to generate the hash. If hmac256 were true, the HMAC-SHA256 algorithm would have been used, otherwise, the JSHash function would be used to generate the hash.
Whenever the user introduces a secret key or new text, the setText, setString, and setSecretKey functions are used to update the variables text, stringText, and secretKey, respectively.
1
2
3
4
5
6
7
const sha256=()=>{
setHmac256(false)
JSHash(text, CONSTANTS.HashAlgorithms.sha256)
.then(hash =>
setString(hash))
.catch(e => console.log(e));
}
This code introduces a function sha256. It uses the JSHash function to create an SHA-256 hash.
sha256 first sets the hmac256 to false, indicating that the JSHash function will be used to build the hash.
It then specifies the function JSHash with two arguments: the text state variable and the CONSTANTS.HashAlgorithms.sha256 constant. It indicates that the SHA-256 hash algorithm will be used.
The JSHash function returns a Promise. It resolves with the created hash value. Further, the function updates the stringText state variable with the new hash value.
Finally, the console.log function is used to log the error to the console, if there is an error during the hashing process.
1
2
3
4
5
const HmacSHA256=()=>{
JSHmac(text, secretKey, CONSTANTS.HmacAlgorithms.HmacSHA256)
.then(hash => setString(hash))
.catch(e => console.log(e));
}
The code snippet defines a HmacSHA256 in the form of a function. It calculates the HMAC using the SHA-256 algorithm on a given text and secretKey.
The JSHmac() function used in the code snippet is a custom implementation of the HMAC algorithm. It uses three parameters namely text, secretKey, and CONSTANTS.HmacAlgorithms.HmacSHA256.
If the JSHmac() is successful in creating the HMAC hash, the new hash value is provided to a setString() function. Console.log() is used to log the error if any.
1
2
3
4
5
6
const MD5=()=>{
setHmac256(false)
JSHash(text, CONSTANTS.HashAlgorithms.md5)
.then(b => setString(b))
.catch(er => console.log(er))
}
It specifies a function MD5 that calculates the MD5 hash of a given text message.
The setHmac256(false) function is called to set the hash function mode to MD5.
If the JSHash() function is successful in creating the MD5 hash, the output (hash value) will be passed to a setString(). This is to set the hash value to some variable or property that can be used later.
1
2
3
4
5
6
const SHA_1=()=>{
setHmac256(false)
JSHash(text, CONSTANTS.HashAlgorithms.sha1)
.then(b => setString(b))
.catch(er => console.log(er))
}
This code snippet defines a function SHA_1. It calculates the SHA-1 hash of a given text.
It calls the setHmac256(false) function before calculating the SHA-1 hash. It is likely that setHmac256() sets some internal state or variable related to the hash calculation process.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
return (
<View>
<TextInput value={text} onChangeText={(prev)=>setText(prev)} style={styles.textInput} placeholder='String....'/>
{hmac256==true?<TextInput value={secretKey} onChangeText={(prev)=>setSecretKey(prev)}
style={styles.textInput} placeholder='SecretKey....'/>:null}
<TouchableOpacity style={styles.hashMac} onPress={()=>sha256()}>
<Text style={styles.Text_header}>sha256</Text>
</TouchableOpacity>
{hmac256==true?<TouchableOpacity style={styles.hashMac} onPress={()=>HmacSHA256()}>
<Text style={styles.Text_header}>HmacSHA256</Text>
</TouchableOpacity>:<TouchableOpacity style={styles.hashMac} onPress={()=>setHmac256(!hmac256)}>
<Text style={styles.Text_header}>HmacSHA256</Text>
</TouchableOpacity>}
<TouchableOpacity style={styles.hashMac} onPress={()=>MD5()}>
<Text style={styles.Text_header}>MD5</Text>
</TouchableOpacity>
<TouchableOpacity style={styles.hashMac} onPress={()=>SHA_1()}>
<Text style={styles.Text_header}>SHA-1</Text>
</TouchableOpacity>
{stringText && <Text style={{color:'#000',marginTop:10}}>{stringText}</Text>}
</View>
)
}
The code renders a text input field to enter a message required to be hashed. It has several buttons for calculating different types of hashes on the message.
When the user enters text into the text input field, the value prop is updated with the new value, and the onChangeText prop updates the state of the text variable with the new value.
The component renders a button to calculate the SHA-256 hash of the message. When users press the button, it will call the sha256() function.
The component also renders a button for getting the HmacSHA256 hash value. If the hmac256 variable is true, it means the user has enabled the HmacSHA256 hash mode. The button will be displayed with a text input field to enter a secret key.
The component further renders buttons for calculating the MD5 and SHA-1 hashes of the message. When the users click these buttons, the MD5() and SHA_1() functions are called, respectively.
After this, the code needs to export the main function HashToken and use the stylesheet to design the component. You can check the code snippet in the code source.
Codebase for the App.js folder
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
import { StyleSheet, Text, View } from 'react-native'
import React from 'react'
import HashToken from './Component/HashToken'
const App = () => {
return (
<View>
<HashToken/>
</View>
)
}
export default App
const styles = StyleSheet.create({})
This code snippet is the entry point for the project. It imports the relevant components, including StyleSheet, Text, View, and HashToken.
The App function defines the main component of the project. It renders the HashToken component wrapped inside a View component.
Finally, the styles object is specified; however, it is empty in this case because no additional styles are needed.
How to validate the accuracy of the build?
The validation can be done by running the program on a device. So, let’s run the program on the emulator. For this, open a terminal from your template folder and pass npm install. Then, you need to pass npx react-native run-android. Running both these commands one after the other will activate the program on the emulator. Refer to image 1 for the final output of this project.
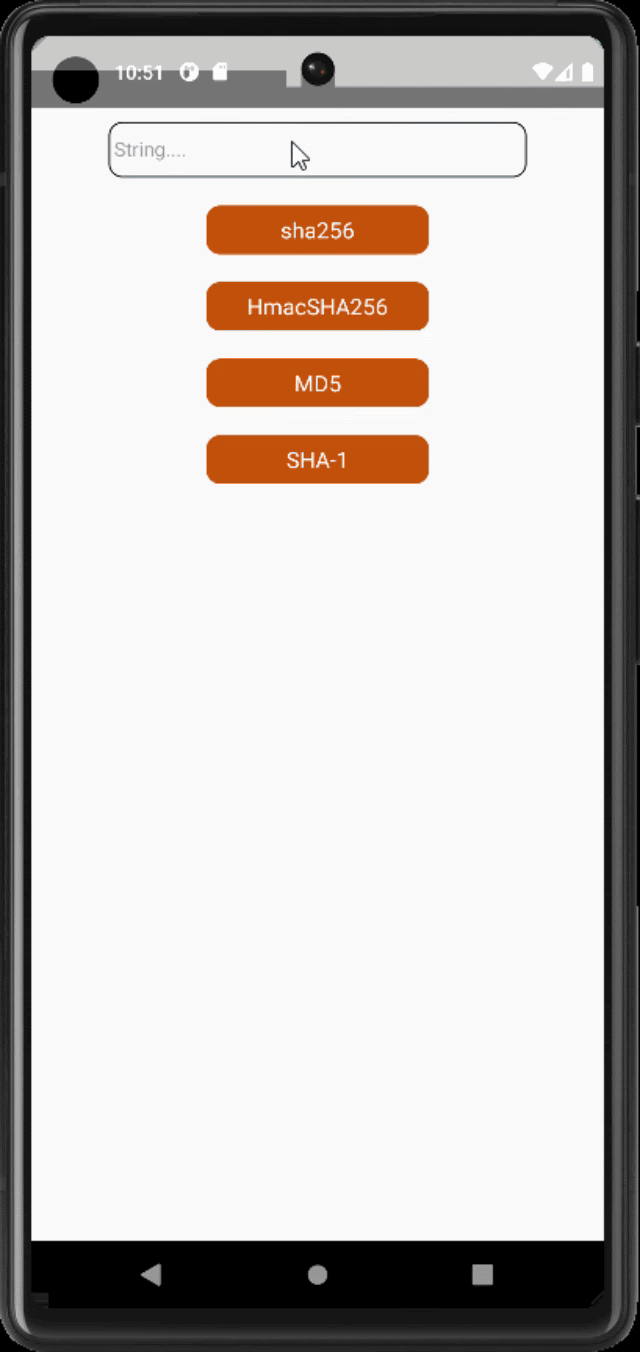
Concluding notes
Hashing is quite a relevant technique in React Native used to generate unique identifiers and comparing data. You can use third-party libraries like react-native-hash to implement hashing in your app and enhance the quality of data management and performance. This blog article is an excellent source to understand the concert and build a real-time hashing app using React Native.

A science graduate who has a keen interest to lean about new technologies and research area. With an experience in the field of data analytics and content writing, she aims to share her knowledge among passionate tech readers.