Unleashing the Power of React Native App Development: Exploring Native Modules and Custom UI
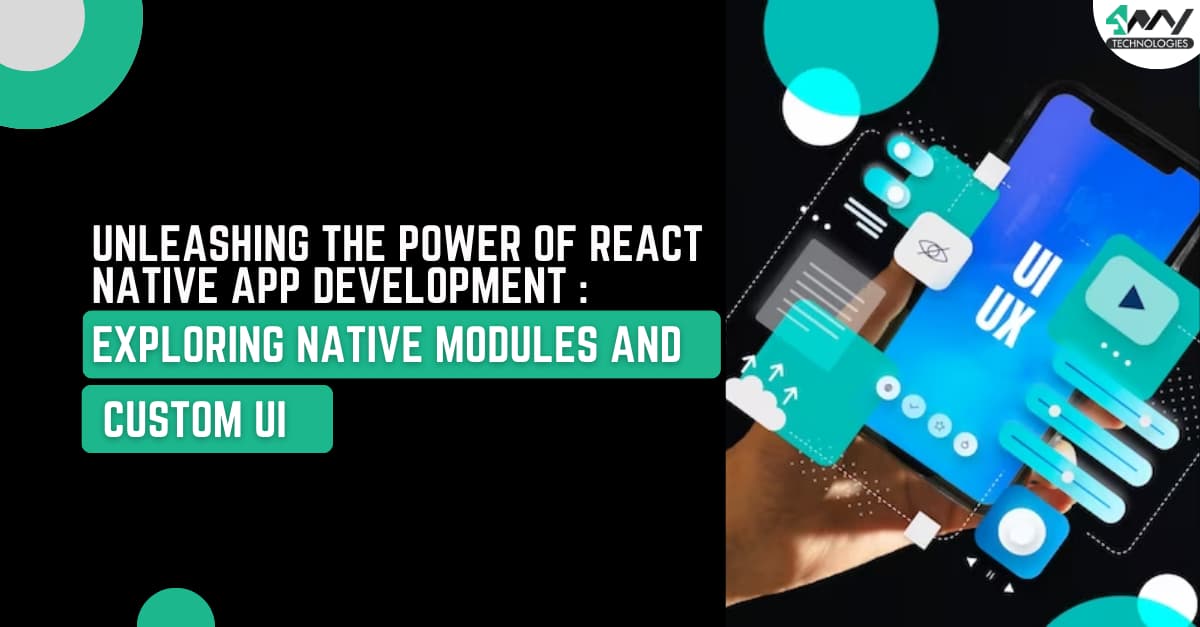
Are you ready to advance your React Native skills? If you have been building React Native apps or are simply curious about how your partner React Native development company is building your app, or even want to add some advanced features or improve the user interface, you have come to the right place! In this blog post, we will delve into the exciting worlds of Native Modules and Custom UI in React Native app development.
Understanding React Native
React Native is a robust framework that enables developers to create cross-platform mobile applications with JavaScript and React. It is well-known for its efficiency and ability to reuse code across platforms, making it a favorite among developers.
However, there may be times when you need to use a device's native capabilities or create a more customized user interface. However, this is where advanced React Native app development techniques come into play.
Native Modules: Bridging the Gap
Native Modules connect the JavaScript code in your React Native app to the native code in Swift (for iOS) or Java/Kotlin (for Android). These modules provide access to native device functionalities that are not available by default in React Native.
Let us take a closer look at Native Modules and how they act as a bridge between the JavaScript code in your React Native app and the device's native code.
Understanding the Bridge
Consider a "bridge" to be a connection that allows two different worlds to communicate. In the context of React Native, there are two worlds: the JavaScript world, where you write the majority of your application logic, and the native world, which contains device-specific functionality.
Native Modules serve as this vital link, seamlessly connecting these two worlds. They act as a mediator, enabling communication between your JavaScript code and the native code written in languages like Swift for iOS or Java/Kotlin for Android.
Why Should You Use Native Modules?
While React Native provides a wide range of cross-platform components, there are times when you need platform-specific functionality. In such cases, Native Modules come in handy, allowing you to access the device's native capabilities.
Consider scenarios in which you want to use a feature that is not readily available through the core components of React Native. Accessing device-specific sensors, leveraging advanced camera functionalities, or integrating with other native APIs are all possibilities. Native Modules allow you to extend the capabilities of your app beyond what React Native provides out of the box.
Accessing Native Functionalities
The native functionalities we are discussing are features unique to the device's underlying operating system. This could involve Swift code for iOS and Java or Kotlin code for Android. Interacting with hardware components, using device sensors, accessing specialized APIs, or performing operations requiring low-level control are examples of such functionalities.
You essentially write the native code required for these functionalities and expose it to your React Native JavaScript code by creating Native Modules. This results in a seamless integration, allowing you to take advantage of the device's full power without sacrificing the cross-platform benefits of React Native.
How Does It Work in Practice?
Creating a Native Module:
When you use a command like npx react-native generate-module MyModule, React Native helps you set up the structure for creating a Native Module.
Implementing Native Code:
The generated files are then opened in your platform-specific IDE (Xcode for iOS or Android Studio for Android) and the native code is written. This could be anything from setting up a camera module to accessing device-specific sensors.
Bridging with JavaScript:
You create a JavaScript interface after writing the native code. This interface serves as a wrapper, allowing your React Native JavaScript code to seamlessly communicate with native functionality.
Integrating and Testing:
Finally, you incorporate your Native Module into your React Native project, import it into your JavaScript code, and begin using it. You gain access to native functionalities through this integration, and you can test your app to ensure everything works as expected.
Native Modules are a powerful tool for any React Native development company because they allow you to combine the best of both worlds: the cross-platform capabilities of JavaScript and React Native, as well as the device-specific functionalities of native code. This bridge enables you to build robust, feature-rich mobile applications that fully utilize the underlying devices.
Getting Started with Native Modules
Creating a Native Module entails a number of steps, but do not worry - it is not as difficult as it sounds! Let's outline the process:
Set Up Your Project:
Check that your React Native project is complete. If you have not already, create a new project with the command:
1
npx react-native init MyProject
Create a Native Module:
Use the following command to generate a new Native Module:
1
npx react-native generate-module MyModule
Implement Native Code:
Once you've generated your Native Module using the command:
1
npx react-native generate-module MyModule
In your preferred Integrated Development Environment (IDE), open the generated native module files. Use Xcode for iOS and Android Studio for Android. Implement the required native code in the files corresponding to the platform on which you are working.
For iOS (Xcode)
- Open the iOS project located at ios/MyProject.xcworkspace in Xcode.
- Navigate to the folder ios/MyModule to find the generated native module files.
- Implement your native code in the relevant Swift files.
For Android (Android Studio)
- Open the Android project located at android in Android Studio.
- Navigate to the folder android/src/main/java/com/MyProject to find the generated native module files.
- Implement your native code in the relevant Java or Kotlin files.
Bridging Native and JavaScript
Follow the steps below to bridge the gap between your native code and JavaScript in React Native:
Define the JavaScript Interface:
Create a JavaScript file, often named MyModule.js, to serve as the interface between your React Native code and the native module.
1
2
3
4
5
6
7
// MyModule.js
import { NativeModules } from 'react-native';
const { MyModule } = NativeModules;
export default MyModule;
Invoke Native Methods:
You can now import and use the methods defined in your native module from within your React Native components.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
// App.js
import React, { useEffect } from 'react';
import MyModule from './MyModule';
const App = () => {
useEffect(() => {
// Call native method
MyModule.myNativeMethod();
}, []);
return (
// Your React Native UI components
);
};
export default App;
Testing Your Module
It is time to test your module now that you have implemented the native code and bridged it with JavaScript.
Integrate into React Native Project:
Check that your JavaScript file has been integrated into your React Native project and that the native code changes have been reflected.
Test the Module:
Run the project and test the integration in your React Native application. You should now be able to use the native functionality you created.
Custom UI: Tailoring the User Experience
While React Native comes with a number of pre-built components for creating user interfaces, there are times when you want to go above and beyond the basics to create a truly unique and engaging user experience. This is where Custom UI components come into play.
Building Custom UI Components
Identifying the Requirements
Before we begin creating custom UI components, we must consider what we want to change about our app's user interface. This could include anything from buttons to sliders to entire screens. Consider which specific elements you want to make unique and engaging for your users.
Creating a New Component
Once you have decided what you want to change, you can use React Native to create a new component. A component in React Native is similar to a building block for the UI of your app. Here's an example of a basic custom button component:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
// CustomButton.js
importReactfrom'react';
import { TouchableOpacity, Text, StyleSheet } from'react-native';
constCustomButton = ({ onPress, title }) => {
return (
<TouchableOpacity onPress={onPress}style={styles.button}>
<Text style={styles.text}>{title}</Text>
</TouchableOpacity>
);
};
const styles = StyleSheet.create({
button: {
backgroundColor: 'blue',
padding: 10,
borderRadius: 5,
},
text: {
color: 'white',
textAlign: 'center',
},
});
exportdefaultCustomButton;
In this example, we've created a CustomButton component that takes two props – onPress for the button press action and titlefor the button text. You can customize the styling to make it look the way you want.
Adding Native Modules if Needed
Native Modules may be required if your custom UI requires special functionality that React Native does not provide out of the box. For example, if your custom component requires access to the device's camera, you would include a Native Module for camera functionality.
1
2
3
4
5
6
7
// CameraModule.js (Native Module)
import { NativeModules } from'react-native';
constCameraModule = NativeModules.CameraModule;
exportdefaultCameraModule;
In your CustomButton.js file, you can now import and use this native module if your button needs to do something camera-related.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
// CustomButton.js
import React from 'react';
import { TouchableOpacity, Text, StyleSheet } from 'react-native';
import CameraModule from './CameraModule'; // Import the native module
const CustomButton = ({ onPress, title }) => {
const handlePress = () => {
// Use the native module functionality
CameraModule.openCamera();
onPress(); // Trigger the provided onPress function
};
return (
<TouchableOpacity onPress={handlePress} style={styles.button}>
<Text style={styles.text}>{title}</Text>
</TouchableOpacity>
);
};
// ... rest of the component
Testing and Iterating
Now that your custom component is complete, it is time to put it through its paces in various scenarios. Start your React Native application and interact with your custom UI component. If something does not appear or function as expected, return to your component file, make changes, and test again. Iterate until your custom UI component has the desired look and functionality. This testing and refining process is essential for creating a great user experience in your React Native app.
Conclusion: Elevate Your React Native Game
You have opened the door to a world of advanced possibilities by investigating Native Modules and Custom UI in React Native. These techniques enable you and your partner React Native development company to take your React Native app development skills to new heights, whether you are accessing native device features or creating a custom user interface.
Always remember that practice makes perfect. Experiment with various Native Modules and custom UI components to solidify your understanding and improve your ability to build remarkable React Native mobile applications. Happy coding!

A science graduate who has a keen interest to lean about new technologies and research area. With an experience in the field of data analytics and content writing, she aims to share her knowledge among passionate tech readers.