Styling in React Native: A Guide to CSS-in-JS and Design Principles
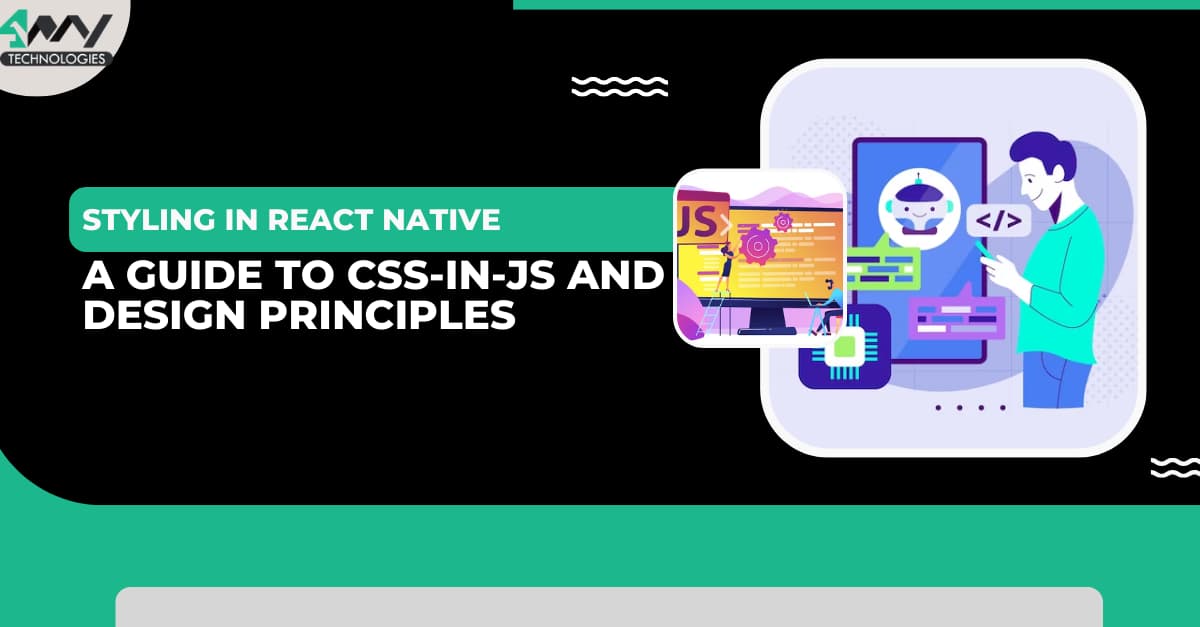
Did you know that 87% of users cite design as a major factor in their decision to use an app? That is right; the appearance of your app can make or break its success. Crafting a visually appealing and user-friendly interface is non-negotiable in the ever-changing app development world.
Understanding the ins and outs of styling when developing React Native apps is a game changer, whether you are a seasoned developer or looking to hire React Native developers. Stay tuned because, in this guide, we will delve into the nuances of CSS-in-JS and key design principles that will set your React Native app apart.
An Overview of React Native Styling
Consider styling in React Native to be a combination of sorcery and simplicity. React Native, unlike traditional web development, has its own way of handling styles to ensure a consistent look and feel across different mobile platforms.
Overview of the React Native Styling Approach
Styles are applied in React Native via a JavaScript-based styling system. We use a subset of CSS properties and values tailored specifically for React Native components instead of CSS. This method enables us to create styles that are compatible with both the iOS and Android platforms.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
import { StyleSheet, View, Text } from 'react-native';
const MyComponent = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, React Native!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 18,
fontWeight: 'bold',
color: 'blue',
},
});
In this example, we are defining styles for our components using the StyleSheet module. Notice how the styles are applied directly to the React Native components, similar to how HTML elements are applied in web development.
Comparison with Web Development Styling
While the fundamental principles may be familiar to web developers, some nuances are unique to React Native. For example, instead of pixels, we frequently use flex to provide layout flexibility. Understanding these distinctions is essential for mastering React Native styling.
Components and Styles
Let us look at how styles are applied to React Native components now. Each component can have its own set of styles, and two approaches are available: default styling and custom styling.
How Styles are Applied:
React Native components are shipped with default styles. A <Text> component, for example, will have default text styling. You can either accept these defaults or modify them to fit the design of your app.
1
2
3
4
5
6
7
8
9
10
import { View, Text } from 'react-native';
const MyComponent = () => {
// Default styling
return (
<View>
<Text>Hello, React Native!</Text>
</View>
);
};
Default Styling vs. Custom Styling:
While default styles are convenient, they may not always match the aesthetics of your app. This is where custom styling comes in. You have full control over the appearance of your components by defining your styles.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
import { StyleSheet, View, Text } from 'react-native';
const MyComponent = () => {
// Custom styling
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, Styled React Native!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
backgroundColor: 'lightblue',
padding: 10,
},
text: {
fontSize: 20,
fontWeight: 'bold',
color: 'darkblue',
},
});
In this example, we used custom styles to modify the background color, padding, font size, as well as the color of the components.
Understanding these fundamentals lays the groundwork for an easy transition into the world of React Native styling. Stay tuned for more in the following sections!
CSS-in-JS in React Native
What is CSS-in-JS?
Explanation of CSS-in-JS Concept:
CSS-in-JS is a styling methodology that incorporates the power of JavaScript into styling. Styles are written directly within JavaScript files rather than in a separate stylesheet. This concept is especially useful in React Native because it allows for dynamic styling and easy integration with component logic.
1
2
3
4
5
6
7
8
9
10
import styled from 'styled-components/native';
const StyledText = styled.Text`
font-size: 18px;
color: ${(props) => (props.isImportant ? 'red' : 'black')};
`;
const MyComponent = ({ isImportant }) => {
return <StyledText isImportant={isImportant}>Hello, CSS-in-JS!</StyledText>;
};
In this example, we used the styled-components library to make a StyledTextcomponent with dynamic styles based on the isImportant prop.
Benefits of Using CSS-in-JS in React Native:
- Dynamic Styling: CSS-in-JS supports dynamic styling based on component props or state, allowing for a more flexible and powerful styling approach.
- Component-based Styling: Styles are encapsulated within components, resulting in a more modular and maintainable code structure.
- Simple Integration with JS Logic: The easy integration with JavaScript logic allows you to manage styles alongside component behavior.
Popular CSS-in-JS Libraries
Overview of Popular Libraries:
Several CSS-in-JS libraries work with React Native, each with its own set of advantages. Styled Components and Emotion are two popular options.
Styled Components
Styled Components is a popular CSS-in-JS library for React and React Native that allows developers to write actual CSS in their JavaScript files, revolutionizing the way styles are applied.
Styled Components simplifies the creation of dynamic and reusable styled elements through its intuitive syntax and component-based styling approach. It promotes a highly maintainable and scalable codebase by providing features such as theming, automatic vendor prefixing, and the ability to easily define responsive styles.
This library improves the developer experience by making React Native styling more powerful and enjoyable.
Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
import styled from 'styled-components/native';
const StyledContainer = styled.View`
flex: 1;
justify-content: center;
align-items: center;
`;
const StyledText = styled.Text`
font-size: 20px;
color: blue;
`;
const MyComponent = () => {
return (
<StyledContainer>
<StyledText>Hello, Styled Components!</StyledText>
</StyledContainer>
);
};
Pros and Cons of Styled Components:
Pros:
- Outstanding developer experience
- Simple syntax
- Styles are seamlessly integrated with component logic.
- Styling components for reusable and maintainable UI elements
- Real-time visual feedback and dynamic styling options
Cons:
- The bundle size has been increased slightly.
- There is a minor trade-off for advantages in developer experience and code maintainability
Emotion
Emotion is a fast and flexible CSS-in-JS library that works well with React and React Native. Emotion is well-known for its emphasis on runtime style composition and offers a powerful API for creating styled-components with minimal overhead.
It provides excellent performance optimizations, such as automatic critical CSS extraction and lazy style loading, which contribute to faster load times. Emotion also supports theming and offers a comprehensive set of features for developing responsive and theme-aware applications.
Emotion stands out as a strong choice for styling in React Native projects due to its emphasis on both developer experience and runtime performance.
Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
/** @jsxImportSource @emotion/react */
import { css } from '@emotion/react';
const containerStyle = css`
flex: 1;
justify-content: center;
align-items: center;
`;
const textStyles = css`
font-size: 20px;
color: green;
`;
const MyComponent = () => {
return (
<View css={containerStyle}>
<Text css={textStyles}>Hello, Emotion!</Text>
</View>
);
};
Pros and Cons of Emotion:
Pros:
- Fantastic performance
- Assistance for the theming
- Style composition at runtime for effective styling
- Automated extraction of important CSS to improve load
- times
Cons:
- Learning curves for some developers at the start
- A rich set of features may take some time to fully comprehend.
- Investing in learning results in improved performance and a more robust styling system.
Implementation Examples
Step-by-step Guide on Implementing CSS-in-JS in React Native:
Install the Library:
1
npm install styled-components
Create a Styled Component:
1
2
3
4
5
6
import styled from 'styled-components/native';
const StyledText = styled.Text`
font-size: 18px;
color: ${(props) => (props.isImportant ? 'red' : 'black')};
`;
Use the Styled Component in Your React Native Component:
1
2
3
const MyComponent = ({ isImportant }) => {
return <StyledText isImportant={isImportant}>Hello, CSS-in-JS!</StyledText>;
};
This step-by-step tutorial will teach you how to integrate CSS-in-JS into your React Native project using the Styled Components library. Experiment with various libraries to find the one that best meets the needs of your project!
Design Principles for React Native Apps
Consistency and Branding
The Importance of Maintaining Consistency in Design
Consistency is the glue that holds a design together, providing users with a consistent and predictable experience. Maintaining visual consistency across components and screens is critical in React Native for creating a polished and professional-looking app. Let us look at how we can accomplish this:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
// Example of consistent styling using a shared theme
// theme.js
export const theme = {
primaryColor: '#3498db',
secondaryColor: '#2ecc71',
fontFamily: 'Roboto',
};
// Usage in components
import { StyleSheet } from 'react-native';
import { theme } from './theme';
const styles = StyleSheet.create({
button: {
backgroundColor: theme.primaryColor,
color: 'white',
borderRadius: 8,
padding: 12,
textAlign: 'center',
fontFamily: theme.fontFamily,
},
});
In this example, we define a theme object that contains design elements that are consistent, such as colors and fonts. This theme is then applied to various components, resulting in a unified visual identity.
How to Incorporate Branding Elements into the App:
Branding elements are extremely important in making your app memorable and recognizable. Integrating these elements harmoniously is critical, whether it is a logo, a specific color scheme, or a unique font. Here's an example of how to include a logo in a React Native app:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
import { Image, StyleSheet } from 'react-native';
import logo from './assets/logo.png';
const Header = () => {
return (
<Image source={logo} style={styles.logo} />
);
};
const styles = StyleSheet.create({
logo: {
width: 120,
height: 40,
},
});
We import a logo image and use it within a Header component in this snippet. By using branding elements consistently throughout your app, you reinforce your brand identity while also creating a more visually engaging user experience.
Responsive Design
Making the App Adaptable to Different Screen Sizes:
Responsive design is critical for providing a consistent user experience across devices and screen sizes. To achieve responsiveness, React Native includes Flexbox, a powerful layout system. Here's an example of a responsive layout made with Flexbox:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
import { View, StyleSheet } from 'react-native';
const ResponsiveLayout = () => {
return (
<View style={styles.container}>
<View style={styles.box}></View>
<View style={styles.box}></View>
<View style={styles.box}></View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flexDirection: 'row',
justifyContent: 'space-between',
},
box: {
width: 80,
height: 80,
backgroundColor: 'blue',
},
});
Flexbox is used in this example to create a row layout that adjusts based on the available space. This ensures that the design is responsive and looks good on both small and large screens.
Methods for Designing a Responsive Layout:
- Flexbox is useful for creating flexible and responsive layouts.
- To accommodate different screen sizes, use percentage-based widths.
- For fine-tuning on specific devices, use media queries or platform-specific code.
3.3 Accessibility
Considering Accessibility When Designing:
Designing with accessibility in mind is more than a best practice; it is a responsibility. When implemented, React Native provides accessibility features that ensure an inclusive user experience. Consider the following scenario:
We use the accessibilityRole and accessibilityLabel props in this snippet to ensure that screen readers correctly identify the text, making it more accessible to users with visual impairments.
Best Practices for Ensuring an Inclusive User Experience:
Provide meaningful accessibilityLabel and accessibilityHint for crucial elements.
Be sure to provide a logical focus order for interactive elements using accessibilityElementsHidden and importantForAccessibility.
Run accessibility tests on your app and collect feedback from users with varying needs.
By implementing these practices, you help to create a more inclusive digital environment, making your React Native app more accessible to a wider audience.
Styling Tips and Tricks
Performance Optimization
Strategies for Optimizing Styling Performance
The performance of any app is critical, and optimizing styling can significantly contribute to a better user experience. To avoid unnecessary recalculations, one common strategy is to use the useMemo hook to memoize styles. Consider the following example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
import { useMemo } from 'react';
import { View, Text, StyleSheet } from 'react-native';
const MyComponent = ({ data }) => {
const containerStyles = useMemo(() => {
return StyleSheet.create({
container: {
backgroundColor: data.isActive ? 'green' : 'red',
padding: 16,
},
text: {
color: 'white',
},
});
}, [data.isActive]);
return (
<View style={containerStyles.container}>
<Text style={containerStyles.text}>Styled React Native Component</Text>
</View>
);
};
We use useMemo in this example to memoize the styles based on the data.isActive prop which prevents unnecessary style recalculations when the component re-renders.
Getting Rid of Unnecessary Re-renders
Re-renders must be kept to a minimum for optimal performance. Use the higher-order component React.memo to memoize functional components and avoid unnecessary re-renders caused by style changes:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
import React, { memo } from 'react';
import { View, Text, StyleSheet } from 'react-native';
const MemoizedComponent = memo(({ text }) => {
return (
<View style={styles.container}>
<Text>{text}</Text>
</View>
);
});
const styles = StyleSheet.create({
container: {
padding: 16,
},
});
By enclosing the component in React.memo, it only re-renders when its props change, avoiding unnecessary rendering cycles.
Theming
Implementing Theming in React Native Apps
Theming gives your app's design more flexibility and consistency. Using a context provider for theming allows components to adjust dynamically based on the theme selected. Here's an example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
import React, { createContext, useContext, useState } from 'react';
import { View, Text, StyleSheet, TouchableOpacity } from 'react-native';
const ThemeContext = createContext();
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
const ThemedComponent = () => {
const { theme, toggleTheme } = useContext(ThemeContext);
const styles = StyleSheet.create({
container: {
backgroundColor: theme === 'light' ? 'white' : 'black',
padding: 16,
},
text: {
color: theme === 'light' ? 'black' : 'white',
},
});
return (
<View style={styles.container}>
<Text style={styles.text}>Themed React Native Component</Text>
<TouchableOpacity onPress={toggleTheme}>
<Text style={styles.text}>Toggle Theme</Text>
</TouchableOpacity>
</View>
);
};
The ThemeProvider in this example provides the current theme as well as a function to toggle it. The ThemedComponent's styles are adjusted based on the active theme, resulting in a dynamic and customizable theming system.
Debugging Styles
Common Styling Issues and How to Troubleshoot Them
Although styling issues are unavoidable, effective debugging can save you time and frustration. To inspect and modify styles on the fly, use React DevTools or the built-in debugging tools in your browser's developer console. Use the react-native-debugger tool as well for a more comprehensive debugging experience.
1
2
3
4
5
6
7
8
// Example of debugging styles using inline styles
const DebuggableComponent = () => {
return (
<View style={{ borderWidth: 2, borderColor: 'red', padding: 16 }}>
<Text style={{ fontWeight: 'bold', color: 'blue' }}>Debug Me!</Text>
</View>
);
};
In this example, we have used a red border to highlight the View component and a bright blue color to distinguish the Text component during debugging.
You will improve the efficiency and maintainability of your React Native app's styling by incorporating these performance optimizations, theming, and debugging techniques.
Future Trends in React Native Styling
Several trends emerged in 2023 that will have an impact on the future of styling in React Native and mobile app development in general. Among these, the key trends and predictions are:
- New Styling Features: React Native introduced new accessibility, style, and event props, as well as feature enhancements to adapt specific CSS styles.
- TypeScript Integration: TypeScript has quickly become the de facto language for React Native, with advantages such as improved code quality and a better developer experience.
- Advanced UI Libraries: The rise of advanced UI libraries in React Native is expected in the future, allowing developers to create more visually appealing and feature-rich mobile applications.
- Rising Community: Engaging with the React Native community is essential for staying current on the latest trends and techniques in styling and mobile app development.
- AI and Machine Learning Integration: As we look forward, more React Native apps will be linked with AI and machine learning technologies, which will likely influence how styling is approached in mobile app development.
A variety of emerging technologies and trends are expected to influence the future of styling in React Native and mobile app development. Developers who want to use these advancements in their projects will need to stay informed and engaged with the React Native community.
Conclusion
And with that, we have embarked on a journey through the intricate world of styling in React Native, covering everything from CSS-in-JS to design principles and useful tips and tricks! Remember the magic of consistency, the power of theming, and the art of debugging as you continue to refine your app's aesthetic.
Whether you are a seasoned pro or just starting out, mastering React Native styling is like discovering a hidden portal to creating visually stunning and performant mobile apps. And if you are ready to take your project to the next level, think about taking a risk and hire React Native developer! You can turn your ideas into reality and create a React Native app that stands out in the digital landscape with their help.
Go forth and code, my friends, armed with new knowledge and a desire for pixel perfection!

A science graduate who has a keen interest to lean about new technologies and research area. With an experience in the field of data analytics and content writing, she aims to share her knowledge among passionate tech readers.