Statecraft in React Native: Redux vs. Mobx vs. Context API Explained
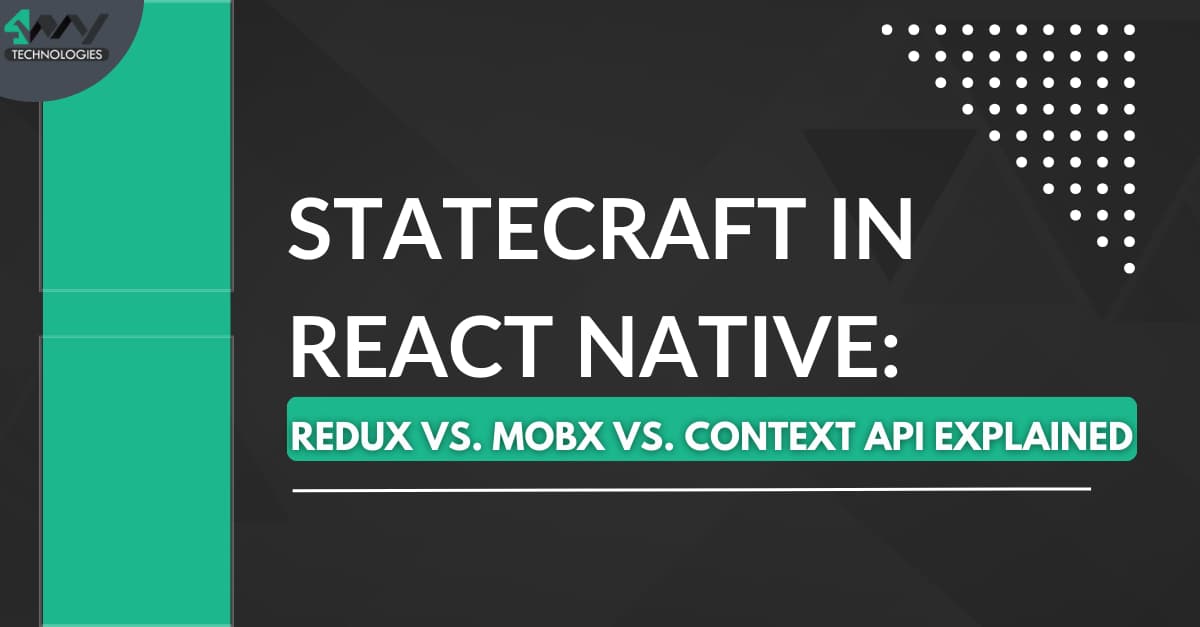
In the vast world of building mobile apps, React Native is a superhero toolkit that has been chosen by over 42% of developers and many a react native development company worldwide.
But here's the thing: as apps become more sophisticated, figuring out how they remember tasks (a.k.a. state management) becomes increasingly important. That is when Redux, Mobx, and the Context API come to the rescue.
Consider Redux to be the Scrum Master with a plan that keeps things predictable and flowing smoothly in large apps. Mobx? More of a laid-back hero, all about simplicity and going with the flow. Then there is the Context API, a built-in React sidekick that keeps things simple for smaller projects.
Which of the three should you choose? It is similar to selecting the best champion for your mission based on the size of your project and the personality of your team. Stay tuned as we explore the capabilities and quirks of Redux, Mobx, and the Context API to help you decide who your app's superhero should be!
Understanding React Native State Management
React Native: The Lego Block Approach to App Development
Consider building a house out of Lego blocks; you have these cool pieces (components) that snap together to create something amazing. That is, in essence, how React Native works! It is similar to having these app building blocks. Instead of starting from scratch, you can use these pre-made pieces (components) to put your app together.
Introducing the MVPs: Components
Everything in React Native is a component—buttons, text boxes, images, you name it. Each of these components functions as a mini Lego block that can be reused and customized. As a result, rather than writing a ton of code for every little thing, you can mix and match these components to create your app. It is like building apps with Legos!
The Function of State: Your App's Memory
Let us now discuss "state." Consider the state to be your app's memory—it remembers things. For example, if your app has a counter that increases when you click a button, the number is saved in the state. It is almost as if your app's brain keeps track of things.
Why State Matter: Issues in Large Apps
Now comes the tricky part. When your app grows into a massive Lego castle with tons of moving parts, managing memory (state) can become difficult. Imagine you have a thousand Lego pieces and you need to know which ones are where—it can be difficult to keep track of them all! Keeping track of all this information and ensuring that everything updates correctly can be difficult in large apps. That is where superhero strategies like Redux, Mobx, or the Context API come in handy to keep our app's brain in check and running smoothly, even when things get massive!
In a nutshell, React Native is like building your app with Legos; state is your app's memory, and as your app grows, it is like managing a giant Lego castle—exciting, but it requires some extra magic to keep everything organized!
Redux
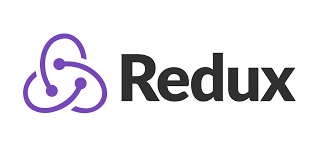
Consider Redux to be a wise master who assists your app in keeping track of things. It is similar to having a box where all of the important information is kept. This is done in accordance with three important rules: actions, reducers, and the store.
- Actions: These are like sticky notes that inform the master about what is going on in your app. When you click a button, you are performing an action! Efficient managers are always interested in what is going on.
- Reducers: These are the people who take action and decide what should happen next. When you press a number-increasing button, the reducer says, "Okay, let us add one!"
- Store: This is the safe place where everything is kept. The store contains all of your app's vital information. At any time, the master can open the box and view the current state of your app.
Unidirectional Data Flow: A One-Way Street
Data flow is a one-way street in Redux. The action begins the journey, passes through the reducer, and finally arrives at the store. It is a tidy process that makes it simple to see how things change in your app.
Pros and Cons: The Good and the Tricky Parts
- Advantages: Redux is ideal for large, complex apps. It organizes everything and makes it simple for developers to understand what is going on. It is similar to having a clear map for your app-adventure.
- Challenges: For small apps, Redux can feel like using a cannon to catch a butterfly at times. It requires some setup, and if your app is not too complex, it may feel like overkill.
- Where Redux Shines: Let us say you have a large app with a lot of components and data. Redux functions similarly to a scrum master, assisting all components in cooperating and ensuring that all data is handled without chaos.
Implementation in React Native: Making Magic Happen
Let us now apply the magic to your React Native app!
Integrating Redux into a React Native Application: A Step-by-Step Guide
Step 1: Install Required Packages
1
npm install redux react-redux
Step 2: Create Actions
Create a file called actions.jsto define your actions.
1
2
3
4
5
6
7
8
// actions.js
export const increment = () => ({
type: 'INCREMENT',
});
export const decrement = () => ({
type: 'DECREMENT',
});
Step 3: Define Reducers
Create a file called reducers.jsto define how your state changes.
1
2
3
4
5
6
7
8
9
10
11
12
13
// reducers.js
const counterReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
export default counterReducer;
Step 4: Create the Store
In your App.js or a separate file, create the Redux store.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
// App.js
import { createStore } from 'redux';
import { Provider } from 'react-redux';
import counterReducer from './reducers';
import AppContainer from './AppContainer'; // Your main component
const store = createStore(counterReducer);
const App = () => (
<Provider store={store}>
<AppContainer />
</Provider>
);
export default App;
Step 5: Connect Components to the Store
In your component (AppContainer.js), connect it to the Redux store.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
// AppContainer.js
import React from 'react';
import { connect } from 'react-redux';
import { increment, decrement } from './actions';
const AppContainer = ({ count, increment, decrement }) => (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
const mapStateToProps = (state) => ({
count: state.count,
});
const mapDispatchToProps = {
increment,
decrement,
};
export default connect(mapStateToProps, mapDispatchToProps)(AppContainer);
Best Practices for Redux Code Structuring in React Native
Organize Files:
- Separate actions, reducers, and the store into their own folders.
- To improve readability, keep related files together.
Use Constants for Action Types:
- To avoid typos and ensure consistency, define action types as constants.
1
2
3
// actions.js
export const INCREMENT = 'INCREMENT';
export const DECREMENT = 'DECREMENT';
Use Middleware Wisely:
- Apply middleware like redux-thunk for handling asynchronous actions.
1
2
3
4
5
6
install redux-thunk
// store.js
import { createStore, applyMiddleware } from 'redux';
import thunk from 'redux-thunk';
import counterReducer from './reducers';
const store = createStore(counterReducer, applyMiddleware(thunk));
You can successfully integrate Redux into your React Native application by following these steps and best practices, resulting in a structured and maintainable codebase for managing your app's state.
Mobx
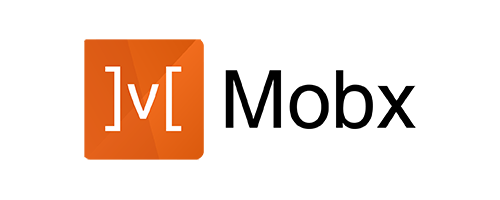
Meet Mobx, your laid-back state management colleague. Mobx has this fantastic philosophy of keeping things simple, similar to how an office should be where everyone can find what they need without feeling stressed.
Observable State: The Office Whiteboard
The state in Mobx is analogous to a whiteboard in an office. When something in your app changes, Mobx automatically notes it on the whiteboard. Assume you are updating a project status; Mobx makes sure everyone is aware of it without you having to go around telling everyone.
Actions and Reactions: Office Tasks and Post-Its
Mobx actions are similar to tasks on a to-do list. When you complete a task, Mobx ensures that the state is updated. Reactions are similar to post-it notes in that they are triggered when the whiteboard (state) changes. So, if the budget changes, Mobx will notify the finance team to double-check the figures!
Compared with Redux: Office vs. Library
Consider Redux to be a well-organized office library. It is great for large projects, but even for small tasks (like checking out a book), you have to go through several steps. Mobx, on the other hand, is like having a direct line to the office organizer—it is quick, simple, and ideal for smaller projects.
Pros and Cons: Mobx - Your Reliable Office Assistant
Advantages: Mobx - Your Office Organizing Pro
- Simplicity: Mobx is like having an office assistant who understands your needs without the need for a manual.It is simple to use and does not overcomplicate things.
- Flexibility: Do you need to reorganize your office (change states)?Mobx is adaptable and will easily adapt to your needs. It is like having a flexible office that can quickly adapt to different tasks.
Limitations: Consider Mobx for the Right Job
- Learning Curve: While Mobx is user-friendly, there may be a learning curve, especially if you are used to different ways of organizing your office (state).
- Best for Smaller Apps: Mobx is ideal for small to medium-sized projects.In case your app is massive, you might prefer Redux's structured shelves.
Use Cases: When Mobx Shines Bright
Small Projects: For those cozy, smaller projects where you need to keep things organized quickly and easily.
Real-Time Updates: If your app requires real-time updates with minimal overhead, Mobx is your go-to assistant.
In the end, Mobx is like your trusted office assistant, keeping things organized, reacting to changes, and simplifying work without adding unnecessary complications. Although it lacks Redux's large library-like structure, Mobx is the go-to organizing tool for the right project
Step-by-Step Guide to Integrating Mobx into a React Native Application:
Step 1: Install Required Packages
1
npm install mobx mobx-react
Step 2: Create a Mobx Store
Create a file named mobxStore.js to define your Mobx store.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
// mobxStore.js
import { observable, action, reaction } from 'mobx';
class CounterStore {
@observable count = 0;
@action increment() {
this.count += 1;
}
@action decrement() {
this.count -= 1;
}
}
const counterStore = new CounterStore();
// Reaction example - reacting to count changes
reaction(() => counterStore.count, (count) => {
console.log(`Count changed to: ${count}`);
});
export default counterStore;
Step 3: Connect Mobx Store to React Native Components
In your component (AppContainer.js), connect it to the Mobx store.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
// AppContainer.js
import React from 'react';
import { observer } from 'mobx-react';
import counterStore from './mobxStore';
const AppContainer = observer(({ count, increment, decrement }) => (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
));
export default AppContainer;
Best Practices for Organizing Mobx Stores and Connecting Components:
Organize Stores:
- Place Mobx stores in a separate folder.
- Create different stores for different parts of your app to separate concerns.
Use the @observable Decorator:
- Use @observable to identify properties that should be monitored.
- This ensures that Mobx tracks changes to these properties.
1
2
3
4
5
// mobxStore.js
import { observable } from 'mobx';
class TodoStore {
@observable todos = [];
}
Actions for State Changes:
- Use@action decorator to define methods that modify the state.
- This ensures that any changes to the state are tracked by Mobx.
1
2
3
4
5
6
7
import { action } from 'mobx';
class TodoStore {
@observable todos = [];
@action addTodo(todo) {
this.todos.push(todo);
}
}
Connect Components with observer:
- Use the observer function frommobx-react to wrap your components.
- This ensures that when the observed data changes, the components re-render.
1
2
3
4
5
// AppContainer.js
import { observer } from 'mobx-react';
const AppContainer = observer(({ count, increment, decrement }) => (
// Component code
));
Reactions for Side Effects:
- Use Mobx reaction for side effects that are not directly related to rendering.
- This is useful for logging, analytics, or any action triggered by state changes.
1
2
3
4
5
// mobxStore.js
import { reaction } from 'mobx';
reaction(() => counterStore.count, (count) => {
console.log(`Count changed to: ${count}`);
});
Ultimately, Mobx makes React Native development easier by providing a simple structure for observable states, actions, and reactions.
Context API
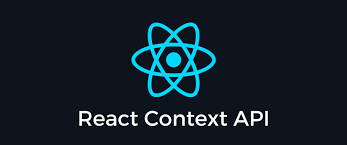
Assume you work in a large office building and need a way to communicate important information to everyone. That is where the React Context API comes in, acting as an office announcement system, allowing you to share updates across the entire workspace without having to run around.
Provider and Consumer Components: The Announcer and Listeners
Consider the Provider to be the official office announcer in this analogy. When something significant occurs (a state change), the announcer informs everyone. On the other side, theConsumer components are like coworkers who listen to the announcements. When they hear the news, they can react or do something.
Simplicity and Lightweight Nature: Communication Made Easy
The beauty of the Context API is its simplicity. It is similar to having a simple intercom system—no complicated setup, just a quick way to share news. It is also lightweight, so it will not clog up your app with unnecessary features.
Pros and Cons: React Context API - The Communication Choice
Advantages: Consistent Communication
- Simplicity: Using the Context API is similar to having a basic office intercom system. It is simple to set up and use, making it simple to communicate between different parts of your office (components).
- Built-in: The Context API is included with React, so no additional packages are required. It is like having a pre-installed office-wide communication tool.
Limitations: Choose Wisely for the Best App Scenario
- Stuck to One Office Floor: The Context API is best suited for smaller offices (components) on the same floor.If your office has multiple floors (complex state management requirements), you might want to consider a more comprehensive system like Redux.
- Too Much Noise for Everyone: Broadcasting announcements to the entire office (using Context everywhere) may result in too much noise for everyone. It is like announcing every detail over the intercom when only a few people need to hear the news.
Performance Considerations: Light on Resources, But...
- Lightweight: The Context API is lightweight and will not add unnecessary features to your app.It is like having a quick communication tool that does not take up too much space.
- But... Keep It Local: Using the intercom system for everything may have an impact on performance. This is not the most efficient way to communicate, similar to sending office-wide announcements for small team updates.
The React Context API is essentially your built-in office announcement system, making communication between different parts of your components simple and straightforward. While it's perfect for smaller apps and simple updates, think twice if your app requires a more extensive communication network or if you're okay with announcing every little detail to everyone.
Implementation in React Native
Step-by-Step Guide to Leveraging the Context API for State Management:
Step 1: Create a Context
Create a file calledMyContext.js to define your context.
1
2
3
4
5
6
// MyContext.js
import { createContext } from 'react';
const MyContext = createContext();
export default MyContext;
Step 2: Wrap Your App with the Provider
In your App.js or a high-level component, wrap your app with the Provider.
1
2
3
4
5
6
7
8
9
10
11
// App.js
import React from 'react';
import MyContext from './MyContext';
const App = () => (
<MyContext.Provider value={{ /* Your shared state here */ }}>
{/* The rest of your app */}
</MyContext.Provider>
);
export default App;
Step 3: Use the Consumer in Child Components
In any child component where you need access to the shared state, use the Consumer.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
// SomeComponent.js
import React from 'react';
import MyContext from './MyContext';
const SomeComponent = () => (
<MyContext.Consumer>
{context => (
<div>
{/* Use context data here */}
</div>
)}
</MyContext.Consumer>
);
export default SomeComponent;
Best Practices for Optimizing Performance and Avoiding Pitfalls:
Avoid Overusing Context:
- Don't use the Context API for everything. Reserve it for a global state or data that genuinely needs to be shared across many components.
Provider Placement:
- Place the Provider at the highest level possible in your component tree. This ensures all child components have access to the shared state.
1
2
3
4
5
6
7
8
9
// App.js
import React from 'react';
import MyContext from './MyContext';
const App = () => (
<MyContext.Provider value={{ /* Your shared state here */ }}>
<ChildComponent />
</MyContext.Provider>
);
Split Contexts for Different Concerns:
- Consider creating multiple contexts if your shared state has different concerns. This prevents unnecessary updates in components that are unconcerned about specific changes.
1
2
3
4
5
6
// UserContext.js
import { createContext } from 'react';
const UserContext = createContext();
export default UserContext;
Memoize Consumers:
- If you have performance concerns, you can use the React.memo HOC to memoize consumers, preventing unnecessary renders.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
// SomeComponent.js
import React, { memo } from 'react';
import MyContext from './MyContext';
const SomeComponent = memo(() => (
<MyContext.Consumer>
{context => (
<div>
{/* Use context data here */}
</div>
)}
</MyContext.Consumer>
));
export default SomeComponent;
Dynamic Context Value:
- If your context value changes frequently, consider memoizing it to avoid unnecessary re-rendering.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
// App.js
import React, { useMemo } from 'react';
import MyContext from './MyContext';
const App = () => {
const contextValue = useMemo(() => ({ /* Your dynamic shared state */ }), []);
return (
<MyContext.Provider value={contextValue}>
{/* The rest of your app */}
</MyContext.Provider>
);
};
export default App;
Let’s say you have now successfully used the Context API for state management in your React Native application by following these steps and best practices. This approach provides a simple and effective way to share state across components while ensuring optimal performance and avoiding common pitfalls.
Choosing the Right State Management for Your Project
Considerations When Choosing a State Management Solution
Project Complexity
- Redux: Suitable for large and complex applications requiring intricate state management.
- Mobx: Excellent for simpler projects or when simplicity trumps extensive features.
- Context API: Excellent for smaller projects with simple state requirements.
Project Size
- Redux: Scalable for large projects, with a structured approach to state management.
- Mobx: Ideal for medium-sized projects, it provides flexibility without adding unnecessary complexity.
- Context API: Suitable for small to medium-sized projects that prioritize simplicity.
Learning Curve
- Redux: Has a steeper learning curve due to its structured nature and the need to understand concepts such as actions, reducers, and the store.
- Mobx: Relatively simple to learn, especially for developers who are already familiar with JavaScript classes and decorators.
- Context API: Has a gentle learning curve, especially for developers who are already familiar with React's component-based architecture.
Comparative Analysis Based on Use Cases
Redux
- Use Case: Large-scale applications with complex state dependencies, like e-commerce platforms, enterprise solutions, or applications with complicated data flows.
- Strengths: Predictable state management, powerful developer tools, excellent debugging, and a large community.
- Considerations: Overhead for smaller projects, boilerplate code, and potential complexity for simple scenarios.
Mobx
- Use Case: Medium-sized applications that value simplicity and reactivity, such as content-driven apps, dashboards, or dynamic interface projects.
- Strengths: Simplicity, flexibility, and reactivity.Ideal for scenarios requiring a more straightforward approach to state management.
- Considerations: May be overkill for small projects, and there may be a learning curve for developers new to reactive programming.
Context API
- Use Case: Small to medium-sized projects that prioritize simplicity and ease of integration, such as portfolio websites, simple applications, or projects with low state complexity.
- Strengths: Built into React, lightweight, and simple to use. Excellent for scenarios requiring a global state but without the overhead of external libraries.
- Considerations: It does not have as many features as Redux or Mobx and may not scale well for highly complex state management.
Guidance on Using a Combination of State Management Solutions
Hybrid Approach
- Scenario: In large projects, consider using Redux or Mobx for critical state management tasks, while employing the Context API for simpler, local state needs.
- Use Redux to manage global app settings and authentication status, while the Context API manages the state of a specific UI component.
Gradual Transition
- Scenario: If transitioning from one state management solution to another appears difficult, consider a gradual approach.
- Begin by introducing the Context API for new features or components while retaining existing Redux functionality. Evaluate the benefits over time and decide whether a complete transition is required.
Performance Optimization
- Scenario: Improve performance by utilizing the Context API for components that do not require frequent updates and Redux or Mobx for parts of the application that have complex state changes.
- Use Redux to manage the state of a real-time chat feature, while the Context API handles the static content of a user profile.
Conclusion
Finally, our investigation of React Native state management solutions reveals three prominent tools: Redux, Mobx, and the Context API. Each has distinct strengths and considerations, making them suitable for a wide range of project scenarios.
Making an informed decision requires an understanding of the project's size, complexity, and team dynamics. The significance of this decision cannot be overstated, as it profoundly influences React Native development efficiency and application performance. Therefore, developers should meticulously analyze project requirements and carefully weigh the pros and cons of each solution. Or you can simply partner with a React Native development company!
Flexibility is essential, and experimenting with various approaches enables developers to tailor their choices to specific project requirements. By adopting this adaptive mindset and taking into account project complexities, developers empower themselves to create resilient and efficient React Native applications that can withstand real-world demands.

A science graduate who has a keen interest to lean about new technologies and research area. With an experience in the field of data analytics and content writing, she aims to share her knowledge among passionate tech readers.