ReactJS: Element Vs Component
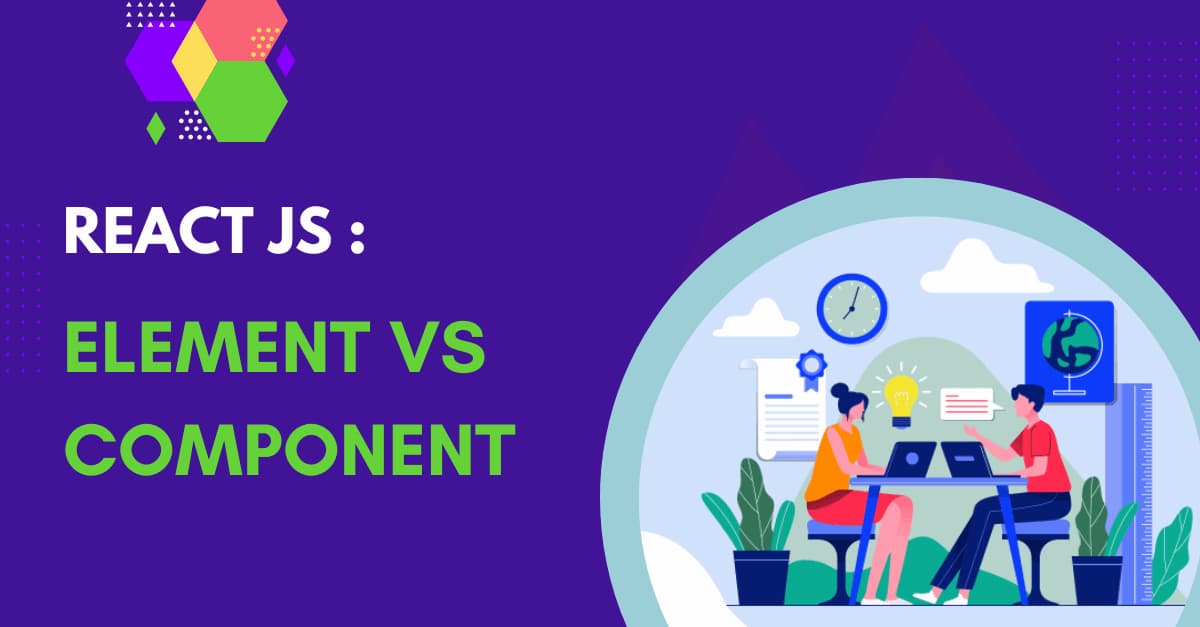
Introduction
Elements and components form the building blocks of the apps you build on ReactJS. These are naturally the most basic concepts that you must gather as you start out working with React, (if not you can always hire ReactJS developers!) Although they both act as structural or functional pieces of your code, elements and components are very different from each other in the way they work. Let us see how.
ReactJS is one of the most powerful libraries that developers use today to build seamless, scalable UIs for applications. The library, hosted by Facebook, is a JavaScript library with several exciting features that sets it apart from other available frameworks. On one hand, ReactJS is dedicated to making app development simpler for us, on the other hand, it ensures that the end products developed on it show the best performance. Features like virtual DOM make apps built on ReactJS highly immersive. Meanwhile, other features, such as reusable components make app development easier for us. This not only saves time and resources but also allows us to be creative while developing apps on React. If you are new to React or have decided to learn React because of these features, you are likely to grasp the basic concepts that form ReactJS. Elements and components in ReactJS are the earliest concepts among these.
Are Elements and Components in ReactJS similar?
No, they aren’t. Components could be said to be the single most important thing to understand or learn in ReactJS. The same could be said about elements in terms of both JavaScript and React. While elements form the building blocks of your codes in ReactJS, components piece together your UI. Components use props as input to render element trees as outputs. Together, they form the very basis of the foundation of your UI built on ReactJS. For this very reason, the concept of elements and components would be among the very first things you learn as a novice to ReactJS or JavaScript. In the beginning, you may find the two to be kind of similar as they are both essential building blocks of your code. They take in data inputs and render actions into the UI display. However, only a slightly closer look would show that they are two very different kinds of pieces in ReactJS.
Elements
In the simplest terms “elements are the smallest building blocks of React apps”. Not to be confused with browser DOM elements, elements in React are plain javascript objects.
Objects are a JavaScript concept. What are objects? Objects are simply entities that have distinct properties and types. Just like a pen is an object that has certain properties like color or shape, and types like roller or fountain, JS objects have properties that define their characters. In JS, examples of an object would be the display of a welcome text, the current date, and so on. Everything in Javascript, except for primitives (like the value of pi), are objects. They are usually a combination of functions, variables, and data structure. We can call objects a group of name values. By being a collection, they increase the readability of our codes.
Creating an Element in React
Elements can be best stated to describe what we want to see on the screen. React virtual DOMs handle the real doms to match React elements. Elements, in turn, make up components. We will discuss this in the next section. Here, let us see an instance of how to create an element in React.
The following element is created using React.createElement(), which takes into account the type of the element, its properties, and children. Here I have created an element consisting of h1 arguments with null properties. A string “Hello Reader” is passed as its children.
1
2
3
4
5
6
7
8
9
10
import React from 'react';
import React from 'react';
import ReactDOM from 'react-dom';
// Without JSX
const ele1 = React.createElement(
'h1',
{id:'header'},
'Hello Learner'
);
ReactDOM.render(ele1,document.getElementById('root'));
The above function would return the following object:
1
2
3
4
5
6
7
{
type: 'h1',
props: {
children: 'Hello Learner',
id: 'header'
}
}
Updating Elements in React
React elements are immutable, once created, their children or attributes cannot be changed. Consider this in the form of an analogy. Elements are like a single frame in a video. We can make changes in the video by removing or replacing frames. Similarly, we can only update elements by creating new elements in their place. It is done by passing the element through root.render()
. This is rather easy in ReactJS as elements are pretty low-cost and simple to create in React. As I said a while ago, virtual DOM compares children and attributes of an element and only applies necessary DOM updates to bring the virtual DOM to the desired state. This helps eliminate bugs as the whole UI does not have to be updated. Changing only one element, basically, like one line of code in the entire component, can update the component.
Let us consider the example of an element that displays the time. To be effective, this element must be updated every second. How to achieve this if elements are immutable? See the following example, the element is updated as root.render()
is called every second from a setInterval() callback
:
1
2
3
4
5
6
7
8
9
10
11
12
13
const root = ReactDOM.createRoot(
document.getElementById('root')
);
function tick() {
const element = (
<div>
<h1>Hello Learner </h1>
<h2>It is {new Date().toLocaleTimeString()}.</h2>
</div>
);
root.render(element);
}
setInterval(tick, 1000);
This functionality can be achieved using State in a class component. We will consider that in the following section.
Components
Components make up the UI of React apps. They are the templates or even, blueprints of the UI. A better way of saying this is that ReactJS allows us to split the UI into components. In this way, individual components of the UI can be manipulated separately. Components operate as independent, reusable pieces.
We can say that components are Javascript functions. They accept arbitrary inputs called props to return react elements to describe what should be displayed on the screen. Components in ReactJS are of two types, namely, function components and class components.
Creating a Function Component
Function components are simple, they are essentially js functions. They accept singular props arguments with data and return a react element. Props stand for properties. Let us see an example of a function component where the prop is a passing user.
1
2
3
4
5
6
7
8
9
import React from 'react';
import ReactDOM from 'react-dom';
function Welcome(user){
return <div>
<h3> Hello {user.name}</h3>
</div>
}
const ele = <Welcome name="Learner"/>
ReactDOM.render(ele,document.getElementById("root"));
Creating a Class Component
Class components use the ES6 class to define a component. Components can be made up of smaller components, as they can be split into smaller components. This helps in extracting and reusing pieces of a component that would be otherwise difficult. This is could be due to the fact that complex components are often ridden with a lot of nesting. Props are read-only. Functions can be pure functions or impure functions. In impure functions, props are variable, but they are fixed in pure functions. In react, a strict thumb rule is that all components must behave like pure functions with respect to props. Dynamic props can be updated using State. State store variables in a single source and allows components to update their props. Let us build a ticking clock class component using state variables.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
class App extends React.Component {
state = {
date: ""
};
getDate() {
var date = { currentTime: new Date().toLocaleString() };
this.setState({
date: date
});
}
render() {
return (
<div class="date">
<p> ddd {this.state.date}</p>
</div>
);
}
}
export default App;
Please note that the name of components must always begin with an uppercase letter. If you use a lowercase letter, react would consider it as a DOM tag.
Differences between Element and Component
At this point, we have a clear comprehension that elements and components in ReactJS are completely different in terms of characters and use. In React, UIs are made of components, which, in turn, are made of elements.
Let us have a quick summary of the key differences between elements and components in React.
Elements | Components |
---|---|
Elements are always returned by components | Components optionally receive inputs to return elements |
Elements are objects without methods | All components have life cycle methods |
Elements are objects that represent individual nodes in the DOM tree | Components form a DOM tree |
Elements are immutable | Components can have mutable states |
React hooks cannot be used with elements as they are immutable | React hooks can be used in functional components |
Elements do not have states and are lighter | Components can have states and are bulkier |
Elements are simpler to create and they render faster | Components are usually complex and render slower than elements |
Summing up
We have come to the end of this discussion where we considered how elements are different from components in ReactJS. While both elements and components act as building blocks, there is a hierarchical difference. Elements together form components and components come together form UIs of React apps. Owing to this, there are some prominent differences in terms of how elements and components are created, and what they consist of. We have summed these up in the above table for easier comprehension. Learning how to create elements and components form the essential basis of creating apps in ReactJS. I hope I managed to clear up any doubts that you might have about the differences between the two.

Sam took the long path into the world of IT. A post-grad in Bioinformatics, she started coding to build better programs for protein sequencing. Currently, Sam actively writes blogs for 4 Way Technologies. She's always excited about all the new technologies and updates in software development. Sam also writes coding tutorials and beginners guides for, well, beginners.