React Native Performance Enhancement: Practices to a Smooth App Experience
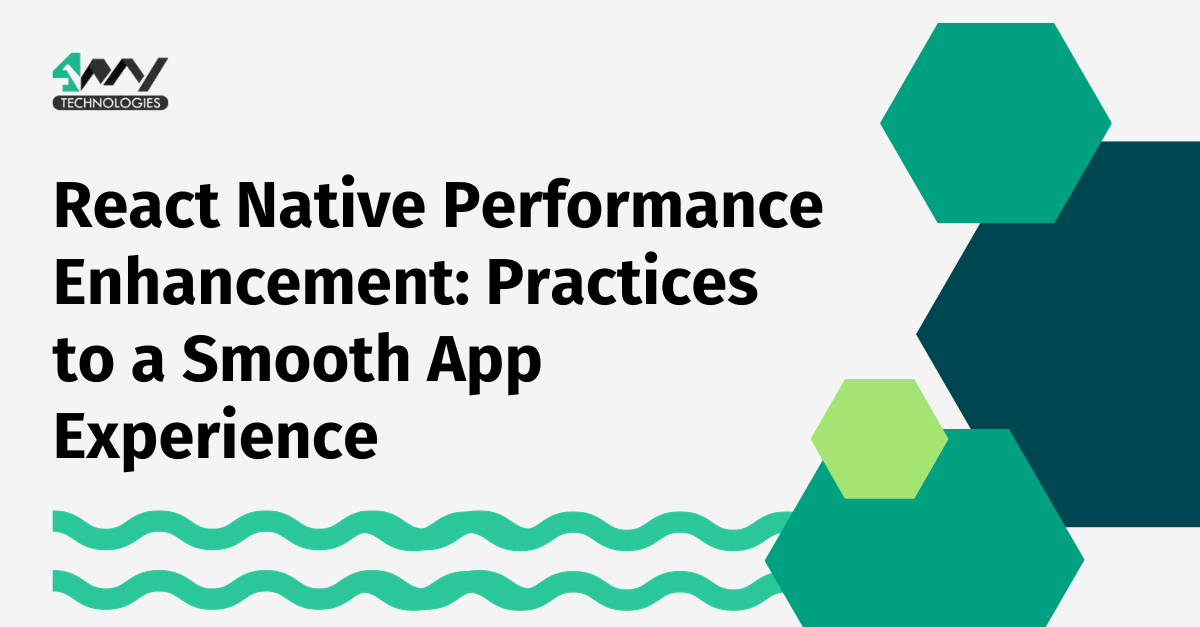
Despite employing efficient coding practices, why does a React Native application sometimes underperform? What differentiates a smooth operating app from one that frequently falters? Do you face the same concern and are stuck in these matters? If yes, you have to work on react native performance optimization. This article will guide you on the way out.
Let’s check the best practices for improving React native performance and enriching user experience from tentative to terrific.
What is the Need for React Native Performance?
Besides user experience, boosting performance in React Native apps will offer you the following benefits.
- To ensure your app stays relevant in this saturated app market.
- Reduces churn rate
- An app and its performance are the reflection of a brand’s credibility. Improving the performance will increase user trust in the brand.
- Improves the discoverability of the app to the target audience.
Interpreting the Bottlenecks For Performance Issues
Dealing with performance issues requires a thorough understanding of what initially caused the problem. Isn’t it? Below are the bottlenecks or sources giving rise to poor React Native performance.
#Problem 1: The most significant cause: JavaScript-Native Bridge
React Native app leverages JS code that is interpreted at runtime. To provide native-like performance, React Native developer needs a communication-based mechanism between the JS and native realm (iOS and Android platforms). This mechanism is the JavaScript-Native Bridge.
But the main question: what is the issue related to this mechanism that hampers the React native performance?
- It is its asynchronous nature. when JavaScript sends a message over the bridge, it doesn’t halt for the previous response to perform. Here, the problems arise if messages are required to be sent frequently.
- Irrelevant use of native modules can lead to multiple calls across the bridge, introducing potential delays and overhead.
- You cannot cross the specified data limit that can be sent across the bridge paper unit of time. Hence, it increases dependency.
#Problem 2: Unnecessary Re-renders in React Native
In React Native app development, a source of performance degradation originates from the negligence of frequent component re-renders. By default, the framework (React Native) triggers a re-render for a component when its props or state undergoes a change. Also, it is applicable for its child components.
This conduct, though efficient, leads to redundant re-renders in scenarios where the state or props remain unchanged. Such a scenario consumes more resources and potentially slows down the app.
#Problem 3: Overburdened JS Thread
If the JavaScript thread becomes overwhelmed with tasks or is forced to handle computationally intensive operations, it can get bogged down. Eventually, problems like slow navigator transitions, delayed user interactions, inconsistent animations, and lagging state updates arise.
Remember that modern apps expect to be responsive and smooth. An overburdened JavaScript thread breaks this expectation.
#Problem 4: The ListView Dilemma Causing Slow List Scrolling
ListView, a React Native component, is used to display data lists. ListView does not utilise the virtualization technique. Hence, it struggles particularly with the initial rendering of large datasets. The component renders every item upon its initiation, which means for large lists, this causes delay.
Since ListView doesn't efficiently handle off-screen items, apps consume excessive memory while presenting long lists. This further leads to app crashes or slowdowns.
#Problem 5: Exaggeration of Console Log Statements
Console statements are used to log output. Possible console statements are ‘console.warn()’, ‘console.log()’
, and console.error()
’. Its use is essential in the development process for identifying issues and debugging code.
However, there lies a major loophole in using such statements. When you run a program in development mode, console logs are used for crucial feedback. But the issue piles up when the app is bundled for production. These log statements remain and continue executing, even though they aren't visible to end-users. This eventually reduces the app's responsiveness and paces down execution.
There are other scenarios that lead to performance issues in React Native. Let’s not focus on the performance glitch and its causes. Instead, prioritize the possible solutions to eliminate performance-based issues in the React Native app.
Practices to Boost React Native Performance
Let’s look for the solutions to the problems discussed in the previous section.
Solution to #Problem 1
- First of all, you can batch or group calls
In this regard, without sending multiple small calls to a native module, batch calls whenever possible. By sending chunks of data or commands, you can reduce the number of interactions or the total number of times data has to cross the bridge.
- Migrate to the TurboModules
After TurboModules were introduced in React Native, developers achieved a streamlined approach addressing react native performance issues due to bridge-related problems.
TurboModules optimises communication between native and JS realms. This way, it reduces the unnecessary back-and-forth across the bridge. The minimised traffic across the bridge ensures efficient app performance.
- Consider a lean structure of data such as JSON data format.
Do you know a leaner data packet translates to faster transmission times, facilitating more rapid communication? The time to serialize and deserialize increases with the size of the data. Hence, go for a more optimized version of data, i.e., JSON format.
This is quite effective in scenarios where you need to exchange high-frequency data, like in real-time updates
Solution to #Problem 2
Employ ‘useMemo’, 'useCallback
', and ‘React.memo
’ if you want to avoid unnecessary re-renders in your React Native apps.
- Consideration of the
useMemo
The useMemo
hook, introduced in React v16.8, manages computations within defined components. Whenever there's a computationally heavy task, useMemo
ensures it's only re-computed when necessary.
For example,
1
2
3
4
5
const expensiveValue = useMemo(() => {
// Your expensive computation mentioned here
}, [dependencies]);
The computation runs only when ‘dependencies’ alter. Otherwise, it returns a cached value.
- Memoizing Functions:
useCallback
Apart from values, functions inside components can also cause re-renders. Use the useCallback hook that returns a memoized version of the callback function. While useMemo returns cached values, useCallback returns cached functions.
For instance,
1
2
3
4
5
const memoizedCallback = useCallback(() => {
// Here is the callback logic
}, [dependencies]);
the inner function of useCallback
only gets executed when the dependencies alter.
- Utilizing the React.memo
As discussed in React v16.6, React.memo
is a Higher Order Component that wraps a component. It ensures that the component only re-renders when its props change.
Here's a basic usage:
const MyComponent = React.memo(function MyComponent(props) {
// your component code goes here
});
Here, MyComponent
re-renders when there is an actual alteration in props. Hence, it is an excellent way to improve performance, particularly in applications with frequent updates.
Solution to #Problem 3
Consider the native driver of React Native with the Animated API.
React Native's Animated API supports serializability. The specifics of an animation can be offloaded to the native side before the animation even starts. Consequently, the animation executes on the UI thread. This occurrence is native and is isolated from the JavaScript thread. This segregation ensures that your animation remains fluid even if the JavaScript thread is burdened with other tasks.
For instance, consider an animation that withers a view from complete opacity to transparent.
When you don’t use the native driver
1
2
3
4
5
6
7
Animated.timing(this.state.opacity, {
toValue: 0,
duration: 1000
}).start();
Whereas when you use the native driver.
1
2
3
4
5
6
7
8
9
Animated.timing(this.state.opacity, {
toValue: 0,
duration: 1000,
useNativeDriver: true
}).start();
In the latter scene, you set the useNativeDriver option to true. This hands off the animation to the native side, and you make way for a smoother user experience.
Given the performance issues due to the overloaded JS thread, here are other practices you should take note of.
- Implement Lazy Loading
Lazy loading strategies instead of loading all components or data up front is preferable for better React Native performance. This implies loading only data or components as they're needed. Hence, you reduce the initial load times.
- Incorporate InteractionManager to Prioritise Tasks
The InteractionManager
allows you to postpone tasks until and after animations or interactions. This ensures smooth user interactions, even if tasks are queued up.
- Optimising Data Structures
Avoid deep object copies and instead consider immutable data structures. Libraries like Immutable.js can help manage data without overburdening the JavaScript thread.
Solution to #Problem 4
Lists hold vital importance in the spectrum of app UIs. Imagine a platform without lists or listing product categories. Can you find any? No. right? What scenarios do you expect if such lists don’t perform consistently? You will lose your potential users.
So, instead of the ListViewcomponent, use modern alternatives in React Native: SetionList
and FlatList
. These are the upgrades regarding app utility and performance. They have built-in performance enhancements, allowing developers to spend comparatively less time fixing glitches.
For lists with fixed-height items, specifying the getItemLayout
prop can drastically speed up the list rendering. It helps these components indicate the position of an item without measuring each one, aiding in quicker responsiveness.
These components support the ‘windowing’ mechanism. Rather than rendering every item in a list, these components only render items currently visible on the screen and a few above and below for a smoother scrolling experience.
Solution to #Problem 5
Implement logic in a way that console statements are executed only in a development environment and not when the app is built for production.
Also, integrate tools like the babel-plugin-transform-remove-console
into the build process. This ensures that it automatically removes all console
.* calls from the production version of the app. Hence, you achieve a satisfactory performance.
Lastly, don't skip a regular review of your codebase. Further, clean up your codebase. Check for unnecessary log statements and get rid of all such statements, especially before moving from development to production.
Other Possible Solutions or Practices
There are other practices that you should consider.
Get Rid of Irrelevant Libraries
Although a basic consideration, it is quite effective. Installing irrelevant libraries or adding assets only makes your React native app element heavy and adds to its overall footprint. It influences the performance of the build. Therefore, make space only for the relevant functionalities and libraries.
Features such as navigation systems, animations, tabs, and others can impact the loading time of screens. The more these elements are present, the greater the potential drag on performance.
Optimizing Image Sizes and Formats
If you are a developer shifting from web to mobile, understand the differences in image handling and its influence on your app performance. While JPG and JPEG formats may be popular for the web due to their compression capabilities, they aren't necessarily the best choice for mobile platforms.
The most performance-friendly image format is WebP, which Google introduced in 2010. Offering lossy and lossless compression modes, WebP decreases image size by 25-34%. Nevertheless, it's worth noting that not all mobile devices support WebP. So, check before you consider this image format.
Furthermore, while web browsers manage images by downloading, scaling, and even caching them efficiently, mobile apps, particularly React Native, handle images differently. React Native's Image component manages individual images well but struggles with multiple large images.
So, what you can do is load images specific to the exact size required. This means resizing and pre-scaling images before adding them to your app.
Harness Firebase Performance Monitoring
You should implement a performance monitoring tool to ensure that your app is performing as per expectation. It's more like a measuring tool that provides timely updates and data insights regarding your app performance.
While the SDK automatically monitors some critical metrics (like app startup time), you can also define custom traces to estimate specific interactions or parts of your app. Most importantly, Firebase provides data from users worldwide, helping you see how your app performs in various regions. This is profitable for brands that particularly want to target a global user base, helping them further optimize the app for regional infrastructures.
Key Takeaways
React Native, although a popular cross-platform app development framework, has major performance bottlenecks that affect the responsiveness and smoothness of the app, leading to poor user satisfaction. Don’t perceive your audience as your friend who will overlook your mistakes even after bringing the matter to your knowledge. The users have a low tolerance and stop interacting with your app if it doesn’t meet their satisfaction.
So, consider the best practices mentioned in this blog for maximizing app performance. This blog highlights several such practices, including image optimization, adopting lean data structures, batching requests, and leveraging the native driver for animations. Of course, the ideal solutions may vary based on the specific performance challenges at hand.

4 Way Technologies is a premium IT services company that develops web, mobile, and SMART TV applications using cutting-edge technologies like React, Node.JS, Deno, Go lang, AWS, and AEM. We establish our thought leadership by sharing content and insights around technology.