Getting Started with React Native: A Comprehensive Beginner's Guide
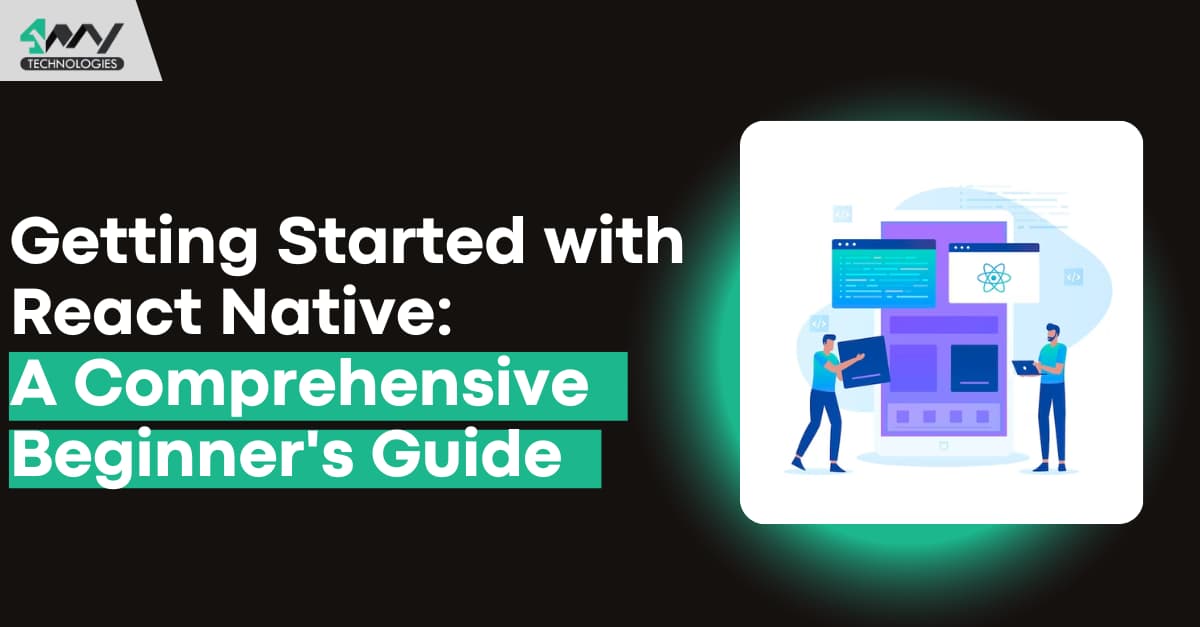
Welcome to the world of React Native development, where the opportunities for building powerful, cross-platform mobile applications are limitless!
If you want to be a React Native developer or are just curious about the exciting world of mobile app development, you have come to the right place. In this comprehensive beginner's guide, we will embark on a journey to demystify React Native and equip you with the necessary skills to launch your career as a skilled React Native developer.
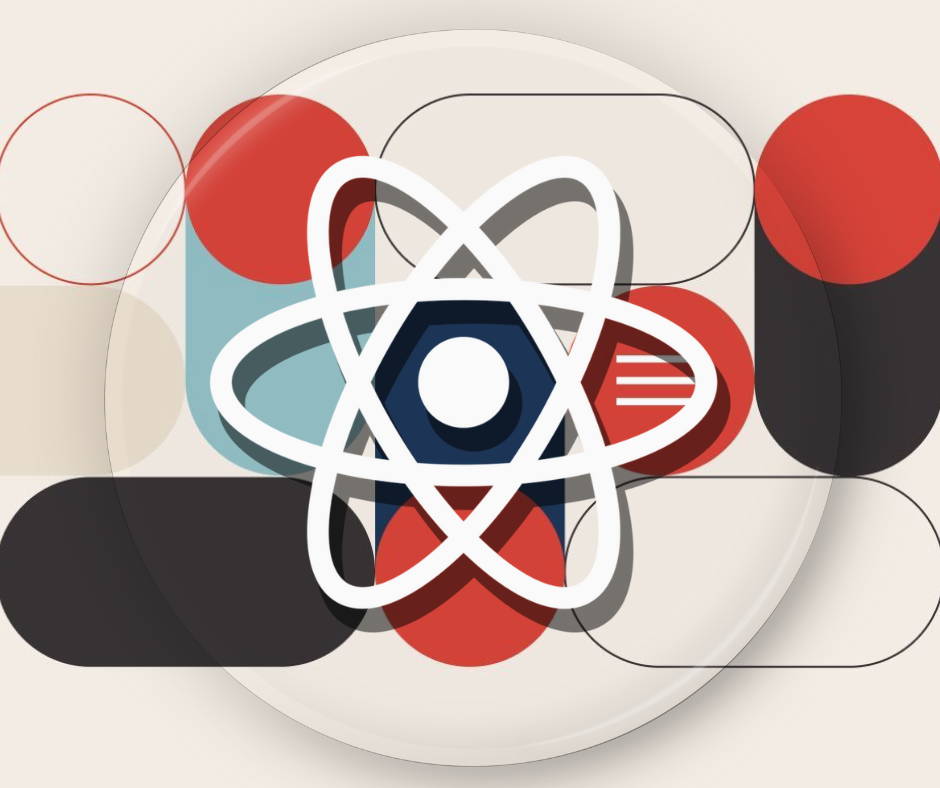
React Native has emerged as a game-changer in today's fast-paced tech landscape, offering a streamlined approach to building mobile applications using the familiar web language: JavaScript.
As a React Native developer, you will be able to create dynamic and high-performance apps for both the Android and iOS platforms while also leveraging your existing web development skills.
This guide is intended for anyone who enjoys coding and wants to learn more about mobile app development. We will walk you through the fundamentals of React Native development, breaking down complex concepts into digestible, practical steps, whether you are a seasoned web developer looking to expand your skill set or a newcomer eager to learn.
So buckle up and prepare for an exciting journey into the heart of React Native. By the end of this guide, you will not only understand the complexities of React Native development, but you will also be well-equipped to create mobile applications and bring your ideas to life in the ever-changing landscape of mobile technology. Let us dive in and discover React Native's full potential together!
Understanding React Native
Definition and Purpose of React Native
Facebook created React Native, an open-source JavaScript framework for developing natively rendered mobile applications. Unlike traditional mobile app development, which frequently necessitates separate codebases for Android and iOS, React Native allows developers to create apps for both platforms using a single codebase. This is accomplished through the use of native components written in Swift (for iOS) and Java or Kotlin (for Android), as well as React components written in JavaScript or TypeScript.
1
2
3
4
5
6
7
8
9
10
11
12
13
// Example of a React Native component
import React from 'react';
import { Text, View } from 'react-native';
const MyApp = () => {
return (
<View>
<Text>Hello, React Native!</Text>
</View>
);
};
export default MyApp;
Comparison with Other Mobile App Development Frameworks
Because of its distinct approach, React Native stands out in the crowded field of mobile app development frameworks. React Native, as opposed to frameworks that rely on WebView components or transpile code to native languages, bridges the gap between native and JavaScript, providing a balance of performance and developer efficiency.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
// Comparison with WebView-based framework (e.g., Cordova)
// React Native
import { WebView } from 'react-native-webview';
const MyWebViewApp = () => {
return (
<WebView source={{ uri: 'https://example.com' }} />
);
};
// Comparison with transpilation-based framework (e.g., Xamarin)
// React Native
import { Text, View } from 'react-native';
const MyXamarinApp = () => {
return (
<View>
<Text>Hello, Xamarin!</Text>
</View>
);
};
The Role of JavaScript in React Native Development
JavaScript is the backbone of React Native, providing the app's logic and functionality. React Native apps follow the same principles as React for the web, allowing developers to create user interfaces with declarative syntax. Furthermore, JavaScript allows for the use of third-party libraries and packages, which expands the capabilities of React Native applications.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
// Example of using JavaScript logic in React Native
import React, { useState } from 'react';
import { Text, Button, View } from 'react-native';
const CounterApp = () => {
const [count, setCount] = useState(0);
return (
<View>
<Text>Count: {count}</Text>
<Button title="Increment" onPress={() => setCount(count + 1)} />
</View>
);
};
export default CounterApp;
Understanding these fundamentals lays the groundwork for delving deeper into React Native development, where you will use React components, native modules, and JavaScript to create engaging and efficient mobile applications.
Setting Up Your Development Environment
Installation of Node.js and npm
Before beginning React Native development, make sure you have Node.js and npm (Node Package Manager) installed on your machine. These are important for managing dependencies and implementing JavaScript code outside the browser.
1
2
3
# Install Node.js and npm using a version manager like nvm
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.0/install.sh | bash
nvm install node
Check the versions of npm and Node.js to confirm the installation:
1
2
node -v
npm -v
Setting up the React Native CLI
For the purpose of starting and maintaining React Native projects, the Command Line Interface (CLI) is an extremely useful tool. A vital first step is to install the React Native CLI globally on your computer.
1
2
# Install React Native CLI globally using npm
npm install -g react-native
Verify the installation:
1
react-native --version
Configuring Your Development Environment for Android and iOS
You will need to set up the required development environments and tools for both Android and iOS in order to create React Native apps for those platforms.
For Android Development:
1
2
3
4
5
6
7
# Install Android Studio (Android development environment)
# Add the following lines to your shell configuration file (e.g., .bashrc or .zshrc)
export ANDROID_HOME=$HOME/Android/Sdk
export PATH=$PATH:$ANDROID_HOME/emulator
export PATH=$PATH:$ANDROID_HOME/tools
export PATH=$PATH:$ANDROID_HOME/tools/bin
export PATH=$PATH:$ANDROID_HOME/platform-tools
For iOS Development:
1
2
3
# Install Xcode (iOS development environment) from the App Store
# Install CocoaPods (dependency manager for iOS projects)
sudo gem install cocoapods
Your development environment is now prepared for the creation of React Native apps with these setups. In the upcoming segments, we will check out how to use these tools to develop and run your first React Native project on emulators or physical devices.
Creating Your First React Native Project
Step-by-Step Guide to Initializing a New React Native Project
Let us use the React Native CLI to create your first React Native project now that your development environment is configured.
1
2
# Create a new React Native project
npx react-native init MyFirstApp
This command downloads the required dependencies and sets up the project structure to begin creating a new React Native project called "MyFirstApp."
Exploring the Project Structure
Explore the project's structure by navigating into the project directory once it has been created.
1
2
# Change directory to your project
cd MyFirstApp
Typically, the project structure looks something like this:
1
2
3
4
5
6
7
8
9
10
MyFirstApp
|-- __tests__/
|-- android/
|-- ios/
|-- node_modules/
|-- .gitignore
|-- App.js
|-- index.js
|-- package.json
|-- README.md
- __tests__/: Folder for your tests.
- android/ and ios/: Native code for Android and iOS respectively.
- node_modules/: Dependencies installed via npm.
- .gitignore: File specifying files and directories to be ignored by version control.
- App.js: Main component file.
- index.js: Entry point of your application.
- package.json: Configuration file for npm dependencies and scripts.
- README.md: Project documentation.
Running Your Project on an Emulator or a Physical Device
Let us now run your React Native project on a real device or an emulator.
1
2
# Run the project for Android
npx react-native run-android
or
1
2
# Run the project for iOS
npx react-native run-ios
Components and Props
Introduction to React Native Components
Components are the fundamental units of the user interface in React Native. It is possible to create intricate interfaces from simpler components by assembling reusable, functional or class-based components.
1
2
3
4
5
6
7
8
9
10
11
12
13
// Example of a functional component
import React from 'react';
import { View, Text } from 'react-native';
const MyComponent = () => {
return (
<View>
<Text>Hello, React Native!</Text>
</View>
);
};
export default MyComponent;
Understanding the Concept of Props
React components receive parameters called props, which is short for properties. They let you dynamically configure and alter components.
1
2
3
4
5
6
7
8
9
10
11
// Example of a component with props
import React from 'react';
import { View, Text } from 'react-native';
const GreetUser = (props) => {
return (
<View>
<Text>Hello, {props.name}!</Text>
</View>
);
};
1
2
// Usage of the component with props
<GreetUser name="John" />
Building and Styling Basic Components
You can use the style prop in React Native to style components. Subsets of the CSS properties are used to define styles.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
// Example of styling a component
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const StyledComponent = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Styled Component</Text>
</View>
);
};
// Styles definition
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#e0e0e0',
},
text: {
fontSize: 18,
color: '#333',
},
});
export default StyledComponent;
State and Lifecycle
Exploring State in React Native
React Native components use states to control and react to changes. It permits interactive and dynamic components.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
// Example of a component with props
import React from 'react';
import { View, Text } from 'react-native';
const GreetUser = (props) => {
return (
<View>
<Text>Hello, {props.name}!</Text>
</View>
);
};
// Usage of the component with props
<GreetUser name="John" />
Building and Styling Basic Components
You can use the style prop in React Native to style components. Subsets of the CSS properties are used to define styles.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
// Example of styling a component
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const StyledComponent = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Styled Component</Text>
</View>
);
};
// Styles definition
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#e0e0e0',
},
text: {
fontSize: 18,
color: '#333',
},
});
export default StyledComponent;
State and Lifecycle
Exploring State in React Native
React Native components use states to control and react to changes. It permits interactive and dynamic components.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
// Example of a stateful component
import React, { useState } from 'react';
import { View, Text, Button } from 'react-native';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<View>
<Text>Count: {count}</Text>
<Button title="Increment" onPress={() => setCount(count + 1)} />
</View>
);
};
export default Counter;
Managing Component Lifecycle Events
A lifecycle is followed by React Native components, from initialization to rendering and updating. Utilizing lifecycle methods, you can carry out tasks at particular stages of this procedure.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
// Example of lifecycle methods in a class component
import React, { Component } from 'react';
import { View, Text } from 'react-native';
class LifecycleComponent extends Component {
componentDidMount() {
console.log('Component did mount');
}
componentDidUpdate() {
console.log('Component did update');
}
componentWillUnmount() {
console.log('Component will unmount');
}
render() {
return (
<View>
<Text>Lifecycle Component</Text>
</View>
);
}
}
export default LifecycleComponent;
Implementing Stateful Components in Your Project
To create dynamic and interactive components for your React Native project, combine the ideas of state and lifecycle. Utilize lifetime methods to carry out operations at various phases of a component's existence and state to manage data that changes over time.
We will cover more complex subjects like user input, navigation, and data fetching in the upcoming segments to help you become a better React Native developer.
Styling in React Native
Overview of Styling Options in React Native
In React Native, styling can be accomplished through style sheets as well as inline objects. JavaScript objects are used to write styles for React Native, which utilizes a subset of CSS properties.
1
2
3
4
5
6
7
8
9
10
11
12
13
// Example of inline styles
import React from 'react';
import { View, Text } from 'react-native';
const InlineStylesExample = () => {
return (
<View style={{ backgroundColor: '#3498db', padding: 20 }}>
<Text style={{ color: '#fff', fontSize: 18 }}>Inline Styles</Text>
</View>
);
};
export default InlineStylesExample;
Using Inline Styles and Style Sheets
While style sheets enable you to create reusable styles and apply them to multiple components, inline styles are defined directly within the component.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
// Example of using style sheets
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const StyleSheetExample = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Styled with StyleSheet</Text>
</View>
);
};
// Styles definition using StyleSheet
const styles = StyleSheet.create({
container: {
backgroundColor: '#27ae60',
padding: 20,
},
text: {
color: '#fff',
fontSize: 18,
},
});
export default StyleSheetExample;
Tips for Responsive Design in Mobile Apps
Your app must have a responsive design in order to adjust to various screen sizes. Flexbox, percentage-based dimensions, and media queries are useful tools for designing layouts that adapt to different screen sizes.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
// Example of a responsive layout using flexbox
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const ResponsiveLayout = () => {
return (
<View style={styles.container}>
<View style={styles.box1}><Text>Box 1</Text></View>
<View style={styles.box2}><Text>Box 2</Text></View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'row',
},
box1: {
flex: 1,
backgroundColor: '#3498db',
alignItems: 'center',
justifyContent: 'center',
},
box2: {
flex: 1,
backgroundColor: '#27ae60',
alignItems: 'center',
justifyContent: 'center',
},
});
export default ResponsiveLayout;
In the upcoming chapter, we'll explore the crucial topic of navigation in React Native, covering different navigation options and setting up a basic navigation structure for your app.
Navigation in React Native
Different Navigation Options in React Native
There are several navigation options available in React Native, such as drawer, tab, and stack navigation. The requirements for the user experience and the structure of your app will determine which navigation strategy is best.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
// Example of stack navigation
import { createStackNavigator } from '@react-navigation/stack';
import Screen1 from './Screen1';
import Screen2 from './Screen2';
const Stack = createStackNavigator();
const StackNavigatorExample = () => {
return (
<Stack.Navigator>
<Stack.Screen name="Screen1" component={Screen1} />
<Stack.Screen name="Screen2" component={Screen2} />
</Stack.Navigator>
);
};
Establishing a Simple Navigation Framework
You can use well-known libraries like React Navigation to set up navigation in React Native. This is an illustration of how to set up a simple stack navigator.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
// Example of setting up a basic navigation structure
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
import HomeScreen from './HomeScreen';
import DetailsScreen from './DetailsScreen';
const Stack = createStackNavigator();
const App = () => {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
};
export default App;
We will go into more detail about passing parameters, customizing the user experience, and navigating between screens in your React Native app in the upcoming sections.
Handling User Input
Capturing User Input with Forms
TextInput, for text input fields, is one of the components offered by React Native that can be used to capture user input.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
// Example of capturing user input with TextInput
import React, { useState } from 'react';
import { View, TextInput, Button } from 'react-native';
const UserInputForm = () => {
const [inputValue, setInputValue] = useState('');
const handleInputChange = (text) => {
setInputValue(text);
};
const handleSubmit = () => {
// Process the input value
console.log('Input Value:', inputValue);
};
return (
<View>
<TextInput
placeholder="Type something..."
onChangeText={handleInputChange}
value={inputValue}
/>
<Button title="Submit" onPress={handleSubmit} />
</View>
);
};
export default UserInputForm;
Validating and Processing User Input
Before processing user input, make sure it satisfies the requirements of your app by validating it.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
// Example of validating and processing user input
import React, { useState } from 'react';
import { View, TextInput, Button, Alert } from 'react-native';
const ValidatedInputForm = () => {
const [inputValue, setInputValue] = useState('');
const handleInputChange = (text) => {
setInputValue(text);
};
const handleSubmit = () => {
// Validate input
if (inputValue.trim() === '') {
Alert.alert('Error', 'Input cannot be empty');
return;
}
// Process the input value
console.log('Input Value:', inputValue);
};
return (
<View>
<TextInput
placeholder="Type something..."
onChangeText={handleInputChange}
value={inputValue}
/>
<Button title="Submit" onPress={handleSubmit} />
</View>
);
};
export default ValidatedInputForm;
Implementing Touch Events and Gestures
React Native enables touch events and gestures via components like TouchableHighlight and TouchableOpacity.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
// Example of touch events and gestures
import React from 'react';
import { View, TouchableOpacity, Text } from 'react-native';
const TouchableComponent = () => {
const handlePress = () => {
// Handle press event
console.log('Button Pressed');
};
return (
<View>
<TouchableOpacity onPress={handlePress}>
<View style={{ padding: 10, backgroundColor: '#3498db' }}>
<Text style={{ color: '#fff' }}>Press Me</Text>
</View>
</TouchableOpacity>
</View>
);
};
export default TouchableComponent;
Fetching Data from APIs
Making API Requests in React Native
Utilize the fetch function or common libraries like axios to create API requests in React Native.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
// Example of making API requests with fetch
import React, { useEffect, useState } from 'react';
import { View, Text } from 'react-native';
const DataFetchingComponent = () => {
const [data, setData] = useState([]);
useEffect(() => {
// Make API request
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
// Handle the fetched data
setData(data);
})
.catch(error => console.error('Error:', error));
}, []);
return (
<View>
{data.map(item => (
<Text key={item.id}>{item.name}</Text>
))}
</View>
);
};
export default DataFetchingComponent;
Handling Responses and Errors
Handle errors and responses from the API to give the user the proper feedback.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
// Example of handling responses and errors
import React, { useEffect, useState } from 'react';
import { View, Text, ActivityIndicator } from 'react-native';
const DataFetchingComponent = () => {
const [data, setData] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
// Make API request
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => {
// Handle the fetched data
setData(data);
setLoading(false);
})
.catch(error => {
console.error('Error:', error);
setLoading(false);
});
}, []);
if (loading) {
return <ActivityIndicator size="large" />;
}
return (
<View>
{data.map(item => (
<Text key={item.id}>{item.name}</Text>
))}
</View>
);
};
export default DataFetchingComponent;
Displaying Data in Your App
After the data has been retrieved, you can use the relevant components to display it in your React Native application.
We will delve into more complex subjects in the following sections, such as deployment, debugging, and navigation in React Native.
Debugging and Testing
Debugging Techniques for React Native
React DevTools, console.log statements, and the platforms' built-in debugging tools—such as React Native Debugger—are some of the tools used for debugging in React Native. This is an illustration using console.log:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
// Example of debugging with console.log
import React, { useEffect, useState } from 'react';
import { View, Text, Button } from 'react-native';
const DebuggingComponent = () => {
const [data, setData] = useState([]);
useEffect(() => {
// Simulate an API request
fetchData();
}, []);
const fetchData = () => {
console.log('Fetching data...');
// Simulate an error
// console.error('Error fetching data');
};
return (
<View>
<Text>Data: {JSON.stringify(data)}</Text>
<Button title="Fetch Data" onPress={fetchData} />
</View>
);
};
export default DebuggingComponent;
Introduction to Testing Tools and Libraries
React Native testing requires the use of tools like Detox for end-to-end testing and Jest for unit testing. It is possible to write unit tests for specific parts or functions.
1
2
3
4
5
6
7
8
9
10
// Example of a simple Jest test
// File: MyComponent.test.js
import React from 'react';
import { render, screen } from '@testing-library/react-native';
import MyComponent from './MyComponent';
test('renders component correctly', () => {
render(<MyComponent />);
expect(screen.getByText('Hello, React Native!')).toBeTruthy();
});
Best Practices for Ensuring Code Quality
By adhering to code style guides and using linters (like ESLint), you can ensure the quality of your code. Here's an instance with ESLint:
1
2
3
4
5
6
7
8
9
// Example ESLint configuration in package.json
{
"eslintConfig": {
"extends": "eslint-config-react-native"
},
"scripts": {
"lint": "eslint ."
}
}
Run the lint script usingnpm run lint to find issues in code quality.
Deployment
Preparing Your React Native App for Deployment
Make sure to set up platform-specific preferences, including app icons, splash screens, and any necessary permissions, before releasing your React Native application.
1
2
// Example of configuring app icons in Android
// File: android/app/src/main/res/mipmap/ic_launcher.png
Building and Packaging Your App for Android and iOS
Create and prepare your React Native application for sharing. For iOS and Android, use these commands:
Android:
1
2
# Build the Android app
cd android && ./gradlew assembleRelease
iOS:
1
2
# Build the iOS app
cd ios && xcodebuild -workspace YourApp.xcworkspace -scheme YourApp -configuration Release
Publishing Your App to App Stores
Creating release builds, submitting them to the appropriate app stores, and adhering to the Google Play Store and Apple App Store guidelines are all part of publishing your app.
Android:
1
2
# Create a release APK for Google Play Store
cd android && ./gradlew bundleRelease
iOS:
1
2
# Create a release build for the App Store
# Open Xcode and follow the steps to create an archive
Once release builds are created, follow the directions in each app store's documentation to submit your React Native application. Make sure you fulfill all the requirements, which include submitting screenshots and app metadata as well as adhering to any review guidelines.
Conclusion
To sum up, this thorough beginner's guide has given you a strong basis on which to build as you begin your career as a React Native developer. By combining the capabilities of JavaScript and React to create effective and captivating applications for both the Android and iOS platforms, React Native development provides an exciting route into the world of cross-platform mobile app creation.
You now understand basic ideas like components, props, state, and lifecycle management as a React Native developer. You now possess the knowledge and abilities necessary to develop dynamic and responsive mobile applications because you have studied the nuances of handling user input, styling, and retrieving data from APIs.
We have talked about the fundamental debugging methods, given you an overview of testing resources and best practices, and emphasized the significance of maintaining code quality as you work on React Native projects. By implementing these procedures into your work process, you will be ready to develop dependable and sturdy applications.
You now know how to build and package your React Native app for iOS and Android, publish it to app stores, and get your app ready for the public during the deployment phase. This last phase is the result of all your hard work; it turns your code into a physical product that can be used by people anywhere in the world.
Always keep in mind that learning is a continuous process as you delve deeper into and progress with React Native development. Remain inquisitive, participate in the active React Native community, and stay up to date on the most recent advancements and industry best practices in this dynamic field. React Native provides a vibrant and fulfilling path for your development career, regardless of whether you are an experienced developer looking to broaden your skill set or a novice eager to influence the direction of mobile applications.
Best of luck on your exciting journey as a React Native developer and congratulations on finishing this guide!

A science graduate who has a keen interest to lean about new technologies and research area. With an experience in the field of data analytics and content writing, she aims to share her knowledge among passionate tech readers.