Exploring the React Native Ecosystem: Essential Libraries and Tools
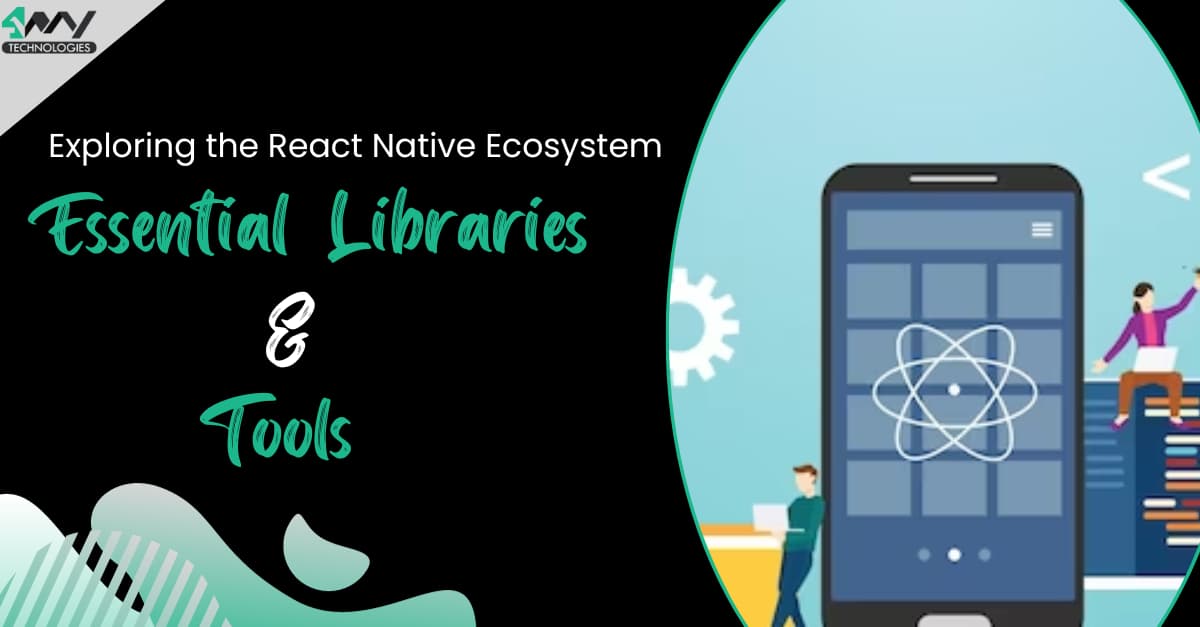
Businesses prefer React Native for cross-platform development because of the unique benefit of using a unified codebase for iOS and Android platforms. This approach results in significant cost savings and a shorter time-to-market, making React Native an appealing option for businesses of all sizes.
React Native is a game-changer in the ever-changing world of mobile app development, reshaping the landscape with its cross-platform capabilities. React Native development services are in high demand, demonstrating their growing popularity among businesses seeking versatile and efficient application solutions.
React Native is more than just a framework; it represents a paradigm shift in how we approach mobile app development. In this blog, we peel back the layers of React Native's libraries and tools, revealing how important they are to the success of React Native application development. Let us navigate the complexities of this ecosystem together, learning how it empowers developers and improves user experiences in an ever-changing tech landscape.
As we embark on a journey through React Native application development, this blog will focus on the critical role that essential libraries and tools play in this framework. The increasing popularity of React Native is not a fluke; it is supported by compelling data demonstrating its cost-effectiveness and optimal performance.
Getting Started with React Native
Setting Up a React Native Project
We use the react-native command-line interface to start a React Native project. Make sure you have Node.js and npm installed before running the following commands:
1
2
3
4
# Install the React Native CLI globally
npm install -g react-native-cli
# Create a new React Native project
react-native init MyAwesomeProject
This will create a basic project structure with all of the required files and folders.
1. Components and JSX
Building components, which are the building blocks of the user interface, are central to React Native development. Here's an example of a simple component:
1
2
3
4
5
6
7
8
9
10
11
12
import React from 'react';
import { View, Text } from 'react-native';
const MyApp = () => {
return (
<View>
<Text>Hello, React Native!</Text>
</View>
);
};
export default MyApp;
2. Styling Components
React Native styling is similar to web development but uses a subset of CSS. Here's an example of how to style a component:
1
2
3
4
5
6
7
8
9
10
11
12
13
const styles = {
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
text: {
fontSize: 20,
textAlign: 'center',
margin: 10,
},
};
Apply styles to the component:
1
2
3
<View style={styles.container}>
<Text style={styles.text}>Styled React Native App</Text>
</View>
Key Features and Advantages
1. Hot Reloading
Hot reloading is supported by React Native, allowing developers to inject new code while keeping the application state intact. Activate it using:
1
2
# Enable Hot Reloading
npm install react-native-reanimated
2. Cross-Platform Development
The fact that React Native is cross-platform is one of its primary advantages. Components like this demonstrate code reusability:
1
2
3
4
5
6
7
8
9
10
11
import { Platform } from 'react-native';
const MyComponent = () => {
return (
<Text>
{`Welcome to ${
Platform.OS === 'ios' ? 'iOS' : 'Android'
} development!`}
</Text>
);
};
Core React Native Libraries
React Navigation
Overview and Significance
React Navigation is a foundational library for managing navigation and routing in React Native apps. Navigation is an important aspect of mobile app development because it allows users to seamlessly switch between different screens or views.
Why is it important?
- Declarative Navigation: React Navigation provides a declarative way to describe your application's navigation structure, making it intuitive and simple to understand.
- Cross-Platform Compatibility: It ensures a consistent navigation experience across both the iOS and Android platforms by adapting to each platform's native navigation patterns.
- Extensibility: React Navigation is extremely extensible, allowing developers to customize and extend navigation behaviors to meet the needs of specific projects.
Implementation and Navigation Patterns
React Navigation supports various navigation patterns, and one of the most common is the Stack Navigator. Here's a basic implementation:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
import { createStackNavigator } from '@react-navigation/stack';
import { NavigationContainer } from '@react-navigation/native';
// Define screens
import HomeScreen from './screens/HomeScreen';
import DetailsScreen from './screens/DetailsScreen';
// Create a Stack Navigator
const Stack = createStackNavigator();
// Wrap your components with NavigationContainer
const App = () => {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
};
export default App;
In this example:
- We importcreateStackNavigatorand NavigationContainer from @react-navigation/stack and @react-navigation/native respectively.
- Define screens (e.g., HomeScreen and DetailsScreen).
- Establish a Stack.Navigator and specify screens using Stack.Screen. The order defines the navigation flow.
This configuration creates a basic stack-based navigation structure, where pushing a new screen onto the stack navigates to the new screen and popping it removes it.
React Navigation also supports other navigation patterns such as Drawer Navigator for slide-in menus, Tab Navigator for tab-based navigation, and others. The best navigation pattern for your app is determined by its structure and user experience goals. React Navigation allows you to combine and customize these patterns to create a navigation flow that is tailored to your application.
Redux for State Management
Why Redux in React Native?
State management is essential in React Native development, and Redux is a well-known library that provides a centralized and predictable state container. Here are some of the reasons why Redux is commonly used in React Native applications:
- Centralized State: Redux centralizes an application's state, making it easier to manage and update. The application's entire state is stored in a single store, making it accessible from any component.
- State Changes Are Predictable: Because Redux enforces a unidirectional data flow, state changes are predictable and traceable. Through reducers, actions cause state changes, making debugging and understanding the application's logic easier.
- Scalability: As the complexity of React Native apps grows, managing state becomes difficult. Redux scales well with larger applications, making it a scalable state management solution.
- Developer Tools: Redux includes powerful developer tools that allow you to inspect and time-travel through state changes. This greatly simplifies debugging and comprehending the application's state evolution.
Integrating Redux in a React Native App
To integrate Redux into a React Native app, follow these general steps:
a. Install Redux and React-Redux:
1
npm install redux react-redux
b. Create Reducers:
To specify how the state changes in response to actions, write reducer functions. For example:
1
2
3
4
5
6
7
8
9
10
11
12
13
// counterReducer.js
const counterReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
export default counterReducer;
c. Create Actions:
Define action creators that return action-descriptor objects.
1
2
3
4
5
6
7
8
// counterActions.js
export const increment = () => ({
type: 'INCREMENT',
});
export const decrement = () => ({
type: 'DECREMENT',
});
d. Create a Store:
Combine reducers and create the Redux store.
1
2
3
4
5
6
7
// store.js
import { createStore } from 'redux';
import counterReducer from './counterReducer';
const store = createStore(counterReducer);
export default store;
e. Connect Components:
Implement the connect function fromreact-reduxto bridge React components to the Redux store.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
import { connect } from 'react-redux';
import { increment, decrement } from './counterActions';
const CounterComponent = ({ count, increment, decrement }) => {
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
const mapStateToProps = (state) => ({
count: state.count,
});
export default connect(mapStateToProps, { increment, decrement })(CounterComponent);
These steps cover the fundamentals of integrating Redux into a React Native app. The actions and reducers can be expanded based on your application's specific state management requirements. The centralized state management provided by Redux aids in the maintenance of a clear and organized structure for state changes in complex React Native projects.
Axios for HTTP Requests
1. Handling API Calls in React Native
Handling API calls is an essential part of developing mobile apps, and Axios is a popular library for making HTTP requests in React Native applications. Here are some of the reasons why Axios is preferred and how API calls can be managed:
- Simplicity and consistency: Axios provides a simple and consistent API for making HTTP requests. It supports promises, making it simple to handle asynchronous operations cleanly and concisely.
- Response and Error Handling: Axios parses JSON responses automatically and provides a convenient way to handle both successful and unsuccessful responses. This streamlines the process of extracting data from responses and dealing with potential problems.
- Interceptors: Axios supports the use of interceptors, which are functions that can be executed globally before, during, or after sending a request or receiving a response. This makes it simple to add custom logic, such as authentication headers, to each request.
- Browser and Node.js Support: Axios is not just for React Native; it can also be used in browser-based and server-side Node.js applications. This adaptability makes it a popular choice for developers working in a variety of environments.
2. Axios Configuration and Use
a. Install Axios:
1
npm install axios
b. Create a Service Module:
Make a separate module to handle API requests. For example:
1
2
3
4
5
6
7
8
9
// apiService.js
import axios from 'axios';
const api = axios.create({
baseURL: 'https://api.example.com', // Replace with your API base URL
timeout: 5000, // Timeout for requests
});
export default api;
c. Make API Calls:
Make API calls in your components or services using the configured Axios instance.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
// Example API call in a React Native component
import React, { useEffect, useState } from 'react';
import api from './apiService';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await api.get('/endpoint');
setData(response.data);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchData();
}, []);
return (
<div>
{data ? (
<p>Data: {JSON.stringify(data)}</p>
) : (
<p>Loading...</p>
)}
</div>
);
};
export default MyComponent;
In this example:
- We establish an Axios instance (api) with a specified base URL and timeout.
- In the React Native component, we choose the useEffect hook to create an asynchronous API call as the component mounts.
- Theapi.get('/endpoint') call collects data from the specified endpoint, and the response is stored in the component's state.
- The component then renders the collected data or a loading message depending on the state.
Encapsulating API calls in a dedicated service module improve code maintainability and reusability. The modular structure enables simple changes to API endpoints and a clear separation of concerns within your React Native application.
AsyncStorage for Local Storage
Storing Data Locally in React Native
Local storage is essential for data persistence in a React Native application, especially when saving user preferences, authentication tokens, or any other data that should persist across app launches. AsyncStorage is a React Native built-in library that provides a straightforward API for asynchronous, persistent key-value storage.
Why AsyncStorage?
- Async Operations: Because AsyncStorage operations are asynchronous, they do not block the main thread and thus have no impact on the app's performance.
- Persistence: Data stored with AsyncStorage is retained even if the app is closed or the device is restarted.
- Key-Value Pair Storage: AsyncStorage is a simple key-value store, making it suitable for storing small amounts of data.
Practical Examples and Best Practices
a. Installing AsyncStorage:
AsyncStorage is included with React Native, so no additional installations are required.
b. Storing Data:
1
2
3
4
5
6
7
8
9
10
11
import AsyncStorage from '@react-native-async-storage/async-storage';
// Storing data
const storeData = async () => {
try {
await AsyncStorage.setItem('username', 'john_doe');
console.log('Data stored successfully!');
} catch (error) {
console.error('Error storing data:', error);
}
};
c. Retrieving Data:
1
2
3
4
5
6
7
8
9
// Retrieving data
const retrieveData = async () => {
try {
const username = await AsyncStorage.getItem('username');
console.log('Retrieved username:', username);
} catch (error) {
console.error('Error retrieving data:', error);
}
};
d. Removing Data:
1
2
3
4
5
6
7
8
9
// Removing data
const removeData = async () => {
try {
await AsyncStorage.removeItem('username');
console.log('Data removed successfully!');
} catch (error) {
console.error('Error removing data:', error);
}
};
Best Practices:
- JSON Serialization: Because AsyncStorage stores values as strings, it is common practice to serialize data as JSON before storing and parse it when retrieving.
1
2
3
4
5
6
7
// Storing a JavaScript object
const user = { name: 'John Doe', age: 25 };
await AsyncStorage.setItem('user', JSON.stringify(user));
// Retrieving and parsing the stored object
const storedUser = await AsyncStorage.getItem('user');
const parsedUser = JSON.parse(storedUser);
- Error Handling: To handle potential errors gracefully, always wrap AsyncStorage operations in try-catch blocks.
- Async/Await Syntax: Use the async/await syntax for readability and to effectively handle asynchronous operations.
- Clearing All Data: Use caution when using clear() to remove all stored data because it will have an impact on the entire app.
1
2
3
4
5
6
7
8
9
// Clearing all data
const clearAllData = async () => {
try {
await AsyncStorage.clear();
console.log('All data cleared successfully!');
} catch (error) {
console.error('Error clearing data:', error);
}
};
By following these practical examples and best practices, you can effectively use AsyncStorage to handle local storage in React Native applications, making sure of data persistence and creating a seamless user experience across app sessions.
UI/UX Libraries
Styled Components
Styling React Native Components
Styling in React Native has traditionally involved using the built-in StyleSheet API, which defines styles using a JavaScript object. Styled-components, on the other hand, provide an alternative approach by allowing developers to write CSS-like styles directly in their React Native components.
Setting up styled-components:
1
npm install styled-components
Using styled-components:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
import styled from 'styled-components/native';
const StyledContainer = styled.View`
flex: 1;
justify-content: center;
align-items: center;
`;
const StyledText = styled.Text`
font-size: 20px;
color: #333;
`;
const MyStyledComponent = () => (
<StyledContainer>
<StyledText>Hello, Styled Components!</StyledText>
</StyledContainer>
);
Benefits of Styled Components
a. Readability and Maintainability:
By encapsulating styles within the component file, styled-components improve code readability. This method provides a clear visual representation of the styling of the component, making it simple to understand and maintain.
b. Dynamic Styling:
Dynamic styling based on props is supported by styled-components, allowing the creation of reusable and flexible components with varying styles.
1
2
3
4
5
6
7
8
9
10
const StyledButton = styled.TouchableOpacity`
background-color: ${(props) => (props.primary ? 'blue' : 'gray')};
padding: 10px;
border-radius: 5px;
`;
// Usage
<StyledButton primary onPress={handlePress}>
<StyledText>Primary Button</StyledText>
</StyledButton>
c. Theming:
Theming is supported by styled-components, making it simple to create light and dark themes, as well as any other theme variations.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
import { ThemeProvider } from 'styled-components/native';
const lightTheme = {
background: '#FFF',
text: '#333',
};
const darkTheme = {
background: '#333',
text: '#FFF',
};
const MyThemedComponent = () => (
<ThemeProvider theme={isDarkMode ? darkTheme : lightTheme}>
<StyledContainer>
<StyledText>Themed Component</StyledText>
</StyledContainer>
</ThemeProvider>
);
d. Global Styles:
Styled-components allow the creation of global styles easily, providing a consistent look and feel across the entire application.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
import { createGlobalStyle } from 'styled-components/native';
const GlobalStyle = createGlobalStyle`
body {
background-color: #F5FCFF;
color: #333;
}
`;
const MyApp = () => (
<>
<GlobalStyle />
{/* Rest of the components */}
</>
);
React Native's styled-components improve the development experience by providing a more intuitive way to style components. It has become a preferred choice for many developers aiming to create elegant and maintainable user interfaces in their React Native applications, thanks to features such as dynamic styling, theming, and improved readability.
React Native Elements
Ready-Made UI Components
React Native Elements is a well-known UI library that offers a collection of ready-made, cross-platform components for building React Native applications. These components include a wide range of UI elements, such as buttons and input fields, as well as more complex components such as cards and sliders.
Installing React Native Elements:
1
npm install react-native-elements
Using Ready-Made Components:
1
2
3
4
5
6
7
8
9
10
11
12
import { Button, Card, Input } from 'react-native-elements';
const MyComponent = () => (
<>
<Card>
<Card.Title>Card Title</Card.Title>
<Card.Divider />
<Input placeholder="Username" />
<Button title="Submit" onPress={handleSubmit} />
</Card>
</>
);
Customization and Usage Tips
a. Customizing Styles:
The style prop is used to customize React Native Elements components. This enables developers to customize the look of components to match the overall theme of the application.
1
2
3
4
5
6
<Button
title="Custom Button"
onPress={handlePress}
buttonStyle={{ backgroundColor: 'purple' }}
titleStyle={{ color: 'white' }}
/>
b. Icons and Icon Placement:
The ability to easily integrate icons into components is included in React Native Elements. Buttons, input fields, and other components can have icons on the left or right side.
c. Theming:
The ThemeProvider component in React Native Elements allows for theming. This ensures that the styling is consistent across all components.
1
2
3
4
5
6
7
8
import { ThemeProvider } from 'react-native-elements';
const MyThemedComponent = () => (
<ThemeProvider>
{/* Components styled according to the theme */}
<Button title="Themed Button" onPress={handlePress} />
</ThemeProvider>
);
Usage Tips:
- Explore the Documentation: React Native Elements has extensive documentation that includes examples and details about each component. Refer to it when working with specific components or investigating customization options.
- Community Support: As a popular library, React Native Elements has an active community. Utilize forums and discussions to seek help or share experiences with other developers using the library.
- Performance Considerations: While React Native Elements is convenient, keep your application's performance in mind. Unused components or excessive customization can slow down the app.
React Native Elements is a great option for developers who want to speed up the UI development process by using pre-built components. It simplifies the creation of aesthetically pleasing and consistent user interfaces in React Native applications by providing customization options, theming support, and a variety of components.
Vector Icons
Integrating Icons in React Native
Icons are essential for improving the visual appeal and usability of mobile applications. Vector Icons is a popular library in the React Native ecosystem for integrating icons into applications.
Vector Icon Installation:
1
2
# Install the required libraries
npm install react-native-vector-icons
Linking Native Modules:
1
2
# Link the native modules (for Android and iOS)
npx react-native link react-native-vector-icons
Using Icons in Components:
1
2
3
4
5
6
7
import Icon from 'react-native-vector-icons/FontAwesome';
const MyComponent = () => (
<View>
<Text>Icon Example: <Icon name="rocket" size={30} color="#900" /></Text>
</View>
);
Popular Icon Libraries and Usage
a. FontAwesome:
FontAwesome is a popular icon set that includes a wide variety of icons for a variety of uses. To use FontAwesome icons with Vector Icons, follow these steps:
1
2
3
4
5
6
7
import Icon from 'react-native-vector-icons/FontAwesome';
const MyComponent = () => (
<View>
<Text>FontAwesome Icon: <Icon name="star" size={30} color="gold" /></Text>
</View>
);
b. Material Icons:
Material Icons, another popular choice for icons, are a part of the Google Material Design system. They are neat and versatile.
1
2
3
4
5
6
import Icon from 'react-native-vector-icons/MaterialIcons';
const MyComponent = () => (
<View>
<Text>Material Icon: <Icon name="android" size={30} color="green" /></Text>
</View>
c. Ionicons:
Ionicons, created by the Ionic framework team, are neat and simple icons great for various applications.
1
2
3
4
5
6
7
import Icon from 'react-native-vector-icons/Ionicons';
const MyComponent = () => (
<View>
<Text>Ionicons Icon: <Icon name="ios-globe" size={30} color="blue" /></Text>
</View>
);
d. Feather Icons:
Feather Icons are a set of beautiful, open-source icons that can be customized. They are light and suitable for a modern and clean user interface.
1
2
3
4
5
6
7
import Icon from 'react-native-vector-icons/Feather';
const MyComponent = () => (
<View>
<Text>Feather Icon: <Icon name="activity" size={30} color="purple" /></Text>
</View>
);
Usage Tips
- Icon Size and Color: Change the size and color of your icons to meet the design and layout needs of your application.
- Consistency: Maintain consistency in icon usage throughout the application to ensure a consistent and professional appearance.
- Custom Icons: Consider using custom icons by importing image assets or exploring other icon libraries if necessary.
- Accessibility: Make sure the icons you choose are easily recognizable and meaningful to users.
Integrating icons with Vector Icons in React Native is a simple process, and the availability of popular icon sets provides developers with a wide range of options to suit the design and theme of their application. When choosing icons for your app, keep the visual style and purpose in mind to create an engaging and user-friendly interface.
Testing and Debugging Tools
Jest for Testing
Setting Up Jest in React Native
Jest is a powerful testing framework that is commonly used for unit and integration testing in React Native projects. Here's a basic setup for using Jest:
Installing Jest:
1
2
# Install Jest and required dependencies
npm install --save-dev jest react-test-renderer @testing-library/react-native
Configuring Jest:
Make a jest.config.js file in the project root or includeJest configuration to package.json:
1
2
3
4
5
6
7
// package.json
{
"jest": {
"preset": "react-native",
"testEnvironment": "node"
}
}
Creating a Test File:
Create tests in files with the.test.js or .spec.js extensions. For instance:
1
2
3
4
5
6
7
8
9
10
// myComponent.test.js
import React from 'react';
import { render } from '@testing-library/react-native';
import MyComponent from './MyComponent';
test('renders correctly', () => {
const { getByText } = render(<MyComponent />);
const textElement = getByText('Hello, Jest!');
expect(textElement).toBeDefined();
});
Writing and Running Tests
Writing Tests:
- Utilize test or it to define a test case.
- Use Jest's expect assertions to test expected outcomes.
Running Tests:
1
2
3
4
5
# Run all tests
npm test
# Run tests in watch mode
npm test -- --watch
Benefits:
- Snapshot Testing: Jest supports snapshot testing, which involves capturing a component's output and comparing it to a previously saved snapshot. This aids in detecting unexpected changes in the user interface.
- Mocking: Mock dependencies or functions to make it easier to isolate the unit of code under test.
- Async Testing: Jest simplifies testing asynchronous code with features such as async/await and Promise handlers.
React Native Debugger
Debugging Techniques in React Native
React Native Debugger is a stand-alone app that combines the functionality of React DevTools and Redux DevTools to provide a powerful environment for debugging React Native applications.
Installing React Native Debugger:
Download and install React Native Debugger from the official GitHub releases.
Debugging Techniques:
- Inspect Element: Right-click on any component to view its properties and current state.
- Redux DevTools: If your app uses Redux, React Native Debugger integrates Redux DevTools seamlessly. Monitor state changes and debug actions.
- Network Inspection: View network requests and responses made by your app.
- Console Logging: Log messages, errors, and warnings directly from the React Native Debugger using the console.
Exploring React Native Debugger Features
a. React DevTools: Use React DevTools to inspect and manipulate the component tree.
b. Redux DevTools: Enable and use the Redux DevTools extension for state management if your app employs Redux.
c. Network Tab:Examine network requests, including their headers and responses.
d. Console:Messages and errors from your application should be recorded.
e. Element Inspector: Examine the UI components and their properties interactively.
f. React Navigation Debugger: If your app makes use of React Navigation, you can inspect and debug navigation state.
Benefits:
- Comprehensive Debugging: The React Native Debugger includes a comprehensive set of debugging tools to make identifying and fixing problems easier.
- Integration with DevTools: The integration of React DevTools and Redux DevTools provides React Native developers with a unified debugging experience.
- Third-Party Integrations: Third-party integrations and plugins are supported by React Native Debugger, allowing for enhanced functionality and customization.
Developers can ensure the dependability and stability of their React Native applications by using Jest for testing and React Native Debugger for debugging. These tools significantly improve the development process by making it easier to write tests, debug code, and gain insights into the behavior of the application.
Performance Optimization
React Native Performance Tips
Identifying and Resolving Performance Bottlenecks
a. React DevTools profiling:
- Profile your components with React DevTools to identify unnecessary renders.
- Determine which components re-render excessively and optimize their rendering logic.
b. Use React Native Performance Tools:
- Tools like react-native-fps-monitor and react-native-performance can help you monitor your app's frame rate and performance.
- Determine which components or operations are causing performance issues and optimize accordingly.
c. Avoid Excessive Re-Renders:
- To avoid unnecessary re-renders, use shouldComponentUpdate or React.memo.
- PureComponent can be used for class components to automatically implement a shallow prop and state comparison.
Best Practices for React Native Performance
a. Virtualization:
- Virtualize long lists with FlatList or SectionList to render only the visible items, improving rendering performance.
b. Image Optimization:
- To control how images are resized, use the resizeMode prop for Image components.
- Consider compressing and optimizing images to reduce their size and improve loading times.
c. Optimizing Bundle Size:
- Reduce the size of your JavaScript bundle by removing unused dependencies and code.
- Using tools like React.lazy and React.Suspence, you can divide your code into smaller chunks for better performance during initial loading.
d. Memoization and Caching:
- In functional components, use libraries like reselect or useMemo to memorize expensive computations or function results.
- To reduce redundant fetches, use caching strategies for network requests and data storage.
e. Native Modules and Components:
- Native modules should be used for CPU-intensive tasks or operations that require native functionality.
- Consider using custom native components for certain performance-critical UI elements.
Continuous Integration and Deployment
Integrating CI/CD in React Native
Automating Builds and Tests
a. Choose a CI/CD Service:
- CircleCI, Travis CI, Jenkins, and GitHub Actions are popular CI/CD services for React Native.
- Choose a service that works well with your version control system (for example, GitHub, GitLab, or Bitbucket).
b. Configure Build Scripts:
- To automate the build process, define build scripts in your project's configuration files (for example, package.json).
- Include commands for installing dependencies, running tests, and constructing the React Native application.
c. Automated Testing:
- Integrate automated testing into your CI/CD pipeline to detect regressions as soon as possible.
- Use unit testing tools such as Jest, end-to-end testing tools such as Deregularlyevant testing libraries.
React Native App Deployment
a. Release and Versioning Branches:
- Use semantic versioning (SemVer) to implement a versioning strategy for your React Native app.
- Release branches should be used for stable versions, while feature branches should be used for ongoing development.
b. Certificates and Code Signing:
- To ensure secure and authorized app distribution, enable code signing on both the iOS and Android platforms.
- Manage iOS certificates and provisioning profiles, as well as Android keystore files.
c. Platforms for Deployment:
- Select platforms for deployment such as the App Store for iOS and Google Play for Android.
- Configure deployment parameters such as app metadata, screenshots, and release notes.
d. Over-the-Air Updates:
- Consider using Over-the-Air (OTA) updates with tools like Microsoft App Center or CodePush to push updates without the need for app store approvals.
e. Continuous Monitoring:
- In production, use continuous monitoring tools to track app performance, crashes, and user behavior.
To gain insights into user engagement, use analytics tools such as Google Analytics or Firebase Analytics.
f. Rollback Strategies:
Create rollback plans in the event that a deployed version introduces critical issues.
After each deployment, track user feedback and app performance to identify potential issues early.
You can automate repetitive tasks, ensure consistent code quality, and streamline the deployment process by incorporating CI/CD practices into your React Native development workflow. Combining effective performance optimization strategies with a solid CI/CD pipeline contributes to your React Native applications' overall success and stability.
Keeping Up-to-Date with React Native Developments
Official Documentation:
Check the official React Native documentation on a regular basis for updates, release notes, and best practices. The documentation provides useful information about new features, changes, and best practices.
Community Forums:
Participate in the React Native community by visiting forums such as GitHub Discussions, Stack Overflow, or the Reactiflux Discord channel. Participating in discussions, asking questions, and sharing experiences with other developers can all provide useful information.
Newsletters and Blogs:
Subscribe to newsletters and blogs that provide updates on React Native, best practices, and emerging trends. Following influential figures and organizations in the React Native community can help you stay up to date on the latest developments.
Conferences and Meetups:
Attend React Native conferences, meetups, and webinars to network with other developers and learn about the latest trends. Events often feature talks, workshops, and presentations by experts in the field.
Social Media:
Follow React Native accounts on social media sites such as Twitter and LinkedIn. Many developers and organizations regularly share React Native development updates, tips, and insights.
Experiment with New Features:
Experiment with new features and updates in small projects or feature branches of existing projects as they are released. Understanding and adapting to changes in the React Native ecosystem requires hands-on experience.
Developers can ensure that they are well-prepared for future trends and updates in React Native development by actively participating in the React Native community, staying informed through official channels, and exploring new features.
Conclusion
Finally, React Native's continuous evolution and the anticipation of upcoming libraries and tools demonstrate its enduring relevance in the dynamic realm of mobile app development. React Native serves as a versatile framework for creating cross-platform applications as developers strive to optimize performance and embrace new features.
React Native development services play an important role in navigating the ever-changing landscape for those seeking expertise, providing tailored solutions for seamless and efficient React Native application development. Adopting these innovations ensures that developers and businesses alike remain at the forefront of innovation in the ever-changing domain of mobile application development

4 Way Technologies is a premium IT services company that develops web, mobile, and SMART TV applications using cutting-edge technologies like React, Node.JS, Deno, Go lang, AWS, and AEM. We establish our thought leadership by sharing content and insights around technology.